Lesson 5. Working with Function Arguments
Learning Objectives
After completing this tutorial, you will be able to:
- Define the purpose of a function argument.
- Use default vs. required function arguments in a function.
What You Need
You will need a computer with internet access to complete this lesson.
In the previous lessons, you have used many different functions and function arguments to customize your code.
For example, you used numerous arguments to plot your data including:
main
to add a title.axes = FALSE
to remove the axes of your plot.box = FALSE
to remove the box surrounding the plot.
In the example below, you call each argument by name and then assign it a value based on the type of argument it is. For example the value for the main =
argument is a text string which is the title that you want R
to add to your plot.
# import and plot landsat
boulder_precip <- raster("data/week-03/BLDR_LeeHill/pre-flood/lidar/pre_DSM.tif")
plot(boulder_precip,
main = "Digital Surface Model for Boulder, Colorado")
Function arguments allow you to customize how a function runs. For example, you use the plot()
function to plot many different types of data. You can use the:
main =
argument to customize the title of the plot.axes =
andbox =
to customize whether R draws the axes and border around the plot.
Plot() is a powerful function as it can be used to do many different things and is customizable in many ways that you may need / want.
Argument Order Matters
You learned this in the first few weeks of class but let’s review it again. The order or arguments supplied to a function matters. R
has three ways that arguments supplied by you are matched to the formal arguments of the function definition:
- By complete name: i.e. you type
main = ""
andR
matches main to the argument calledmain
. - By order or position when you call an argument: i.e. you call
plot(raster, "title here")
,R
will read these two variables in the order that you provide them. This can cause the function to fail if they are not in the right order! - By partial name: (matching on initial n characters of the argument name) - you are not going to review this in this class. Beware using this “feature”.
Arguments are matched in the manner outlined above.
R
first tries to find arguments according to the complete name,- then by partial matching of names,
- and finally by position.
With that in mind, let’s look at the help for read.csv()
:
# view help for the csv function
?read.csv
There’s a lot of information available in the help. The the most important part is the first couple of lines:
read.csv(file, header = TRUE, sep = ",", quote = "\"",
dec = ".", fill = TRUE, comment.char = "", ...)
This tells us that read.csv()
has one argument, file
, that doesn’t have a default value, and six other arguments that do have a default value.
Now you understand why the following code returns an error:
precip_data <- read.csv(FALSE, "data/week-02/precipitation/precip-boulder-aug-oct-2013.csv")
The code above fails because FALSE
is assigned to file
and the filename is assigned to the argument header
.
Default Function Arguments
You have passed arguments to functions in two ways:
- Directly:
plot(landsat_ndvi)
- By name:
read.csv(file = "data/week-02/precipitation/precip-boulder-aug-oct-2013.csv", header = FALSE)
.
You can pass the arguments to read.csv
without naming them if they are in the order that R
expects.
precip_data <- read.csv("data/week-02/precipitation/precip-boulder-aug-oct-2013.csv",
FALSE)
However, the position of the arguments matter if they are not named. Does the code below return an error?
# import csv
precip_data <- read.csv(header = FALSE,
file = "data/week-02/precipitation/precip-boulder-aug-oct-2013.csv")
But this code below doesn’t work. Make sense?
dat <- read.csv(FALSE,
"data/week-02/precipitation/precip-boulder-aug-oct-2013.csv")
Share on
Twitter Facebook Google+ LinkedIn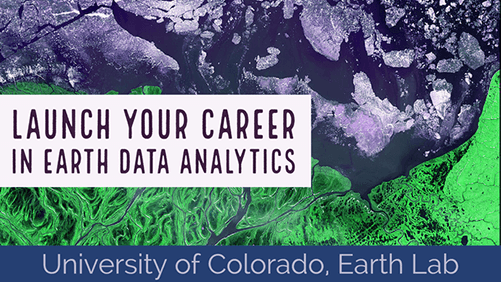
Leave a Comment