Lesson 2. Open and Use MODIS Data in HDF4 format in Open Source Python
Learning Objectives
- Read MODIS data in HDF4 format into Python using open source packages (xarray).
- Extract metadata from HDF4 files.
- Plot data extracted from HDF4 files.
In this lesson, you will learn how to open a MODIS HDF4 format file using xarray.
To begin, import your packages.
# Import packages
import os
import warnings
import matplotlib.pyplot as plt
import numpy.ma as ma
import xarray as xr
import rioxarray as rxr
from shapely.geometry import mapping, box
import geopandas as gpd
import earthpy as et
import earthpy.spatial as es
import earthpy.plot as ep
warnings.simplefilter('ignore')
# Get the MODIS data
et.data.get_data('cold-springs-modis-h4')
# This download contains the fire boundary
et.data.get_data('cold-springs-fire')
# Set working directory
os.chdir(os.path.join(et.io.HOME,
'earth-analytics',
'data'))
Downloading from https://ndownloader.figshare.com/files/10960112
Extracted output to /root/earth-analytics/data/cold-springs-modis-h4/.
Hierarchical Data Formats - HDF4 - EOS in Python
In the previous lesson, you learned about the HDF4 file format, which is a common format used to store MODIS remote sensing data. In this lesson, you will learn how to open and process remote sensing data stored in HDF4 format.
You can use rioxarray to open HDF4 data. Note that both tools wrap around gdal and will make the code needed to open your HDF4 data, simpler.
To begin, create a path to your HDF4 file.
# Create a path to the pre-fire MODIS h4 data
modis_pre_path = os.path.join("cold-springs-modis-h4",
"07_july_2016",
"MOD09GA.A2016189.h09v05.006.2016191073856.hdf")
modis_pre_path
'cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf'
Open HDF4 Files Using Open Source Python and Xarray
HDF files are hierarchical and self describing (the metadata is contained within the data). Because the data are hierarchical, you will have to loop through the main dataset and the subdatasets nested within the main dataset to access the reflectance data (the bands) and the qa layers.
Below you open the HDF4 file. Notice that rioxarray
returns a list rather than an single xarray object. Within that list are two xarray objects representing the two groups in the h4 file.
# Open data with rioxarray
modis_pre = rxr.open_rasterio(modis_pre_path,
masked=True)
type(modis_pre)
list
The first object returned in the list contains all of the quality control layers. Notice that each layer is stored as a data variable.
# This is just a data exploration step
modis_pre_qa = modis_pre[0]
modis_pre_qa
<xarray.Dataset> Dimensions: (y: 1200, x: 1200, band: 1) Coordinates: * y (y) float64 4.447e+06 4.446e+06 ... 3.336e+06 * x (x) float64 -1.001e+07 -1.001e+07 ... -8.896e+06 * band (band) int64 1 spatial_ref int64 0 Data variables: num_observations_1km (band, y, x) float32 ... granule_pnt_1 (band, y, x) float32 ... state_1km_1 (band, y, x) float32 ... SensorZenith_1 (band, y, x) float32 ... SensorAzimuth_1 (band, y, x) float32 ... Range_1 (band, y, x) float32 ... SolarZenith_1 (band, y, x) float32 ... SolarAzimuth_1 (band, y, x) float32 ... gflags_1 (band, y, x) float32 ... orbit_pnt_1 (band, y, x) float32 ... Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- y: 1200
- x: 1200
- band: 1
- y(y)float644.447e+06 4.446e+06 ... 3.336e+06
array([4447338.76595 , 4446412.140517, 4445485.515084, ..., 3338168.122583, 3337241.49715 , 3336314.871717])
- x(x)float64-1.001e+07 ... -8.896e+06
array([-10007091.364283, -10006164.73885 , -10005238.113417, ..., -8897920.720916, -8896994.095483, -8896067.47005 ])
- band(band)int641
array([1])
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -10007554.677 926.625433055833 0.0 4447802.078667 0.0 -926.6254330558334
array(0)
- num_observations_1km(band, y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- Number of Observations
- units :
- none
[1440000 values with dtype=float32]
- granule_pnt_1(band, y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- Granule Pointer - first layer
- units :
- none
[1440000 values with dtype=float32]
- state_1km_1(band, y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- 1km Reflectance Data State QA - first layer
- units :
- bit field
[1440000 values with dtype=float32]
- SensorZenith_1(band, y, x)float32...
- scale_factor :
- 0.01
- add_offset :
- 0.0
- long_name :
- Sensor zenith - first layer
- units :
- degree
[1440000 values with dtype=float32]
- SensorAzimuth_1(band, y, x)float32...
- scale_factor :
- 0.01
- add_offset :
- 0.0
- long_name :
- Sensor azimuth - first layer
- units :
- degree
[1440000 values with dtype=float32]
- Range_1(band, y, x)float32...
- scale_factor :
- 25.0
- add_offset :
- 0.0
- long_name :
- Range (pixel to sensor) - first layer
- units :
- meters
[1440000 values with dtype=float32]
- SolarZenith_1(band, y, x)float32...
- scale_factor :
- 0.01
- add_offset :
- 0.0
- long_name :
- Solar zenith - first layer
- units :
- degree
[1440000 values with dtype=float32]
- SolarAzimuth_1(band, y, x)float32...
- scale_factor :
- 0.01
- add_offset :
- 0.0
- long_name :
- Solar azimuth - first layer
- units :
- degree
[1440000 values with dtype=float32]
- gflags_1(band, y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- Geolocation flags - first layer
- units :
- bit field
[1440000 values with dtype=float32]
- orbit_pnt_1(band, y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- Orbit pointer - first layer
- units :
- none
[1440000 values with dtype=float32]
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
You can access a data variable in a similar fashion to how you would access a column in a pandas DataFrame using the ["variable-name-here"]
.
modis_pre_qa["num_observations_1km"]
<xarray.DataArray 'num_observations_1km' (band: 1, y: 1200, x: 1200)> [1440000 values with dtype=float32] Coordinates: * y (y) float64 4.447e+06 4.446e+06 ... 3.337e+06 3.336e+06 * x (x) float64 -1.001e+07 -1.001e+07 ... -8.897e+06 -8.896e+06 * band (band) int64 1 spatial_ref int64 0 Attributes: scale_factor: 1.0 add_offset: 0.0 long_name: Number of Observations units: none
- band: 1
- y: 1200
- x: 1200
- ...
[1440000 values with dtype=float32]
- y(y)float644.447e+06 4.446e+06 ... 3.336e+06
array([4447338.76595 , 4446412.140517, 4445485.515084, ..., 3338168.122583, 3337241.49715 , 3336314.871717])
- x(x)float64-1.001e+07 ... -8.896e+06
array([-10007091.364283, -10006164.73885 , -10005238.113417, ..., -8897920.720916, -8896994.095483, -8896067.47005 ])
- band(band)int641
array([1])
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -10007554.677 926.625433055833 0.0 4447802.078667 0.0 -926.6254330558334
array(0)
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- Number of Observations
- units :
- none
The second element in the list contains the reflectance data. This is the data that you will want to use for your analysis
# Reflectance data
modis_pre_bands = modis_pre[1]
modis_pre_bands
<xarray.Dataset> Dimensions: (y: 2400, x: 2400, band: 1) Coordinates: * y (y) float64 4.448e+06 4.447e+06 ... 3.336e+06 * x (x) float64 -1.001e+07 -1.001e+07 ... -8.896e+06 * band (band) int64 1 spatial_ref int64 0 Data variables: num_observations_500m (band, y, x) float32 ... sur_refl_b01_1 (band, y, x) float32 ... sur_refl_b02_1 (band, y, x) float32 ... sur_refl_b03_1 (band, y, x) float32 ... sur_refl_b04_1 (band, y, x) float32 ... sur_refl_b05_1 (band, y, x) float32 ... sur_refl_b06_1 (band, y, x) float32 ... sur_refl_b07_1 (band, y, x) float32 ... QC_500m_1 (band, y, x) float64 ... obscov_500m_1 (band, y, x) float32 ... iobs_res_1 (band, y, x) float32 ... q_scan_1 (band, y, x) float32 ... Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- y: 2400
- x: 2400
- band: 1
- y(y)float644.448e+06 4.447e+06 ... 3.336e+06
array([4447570.422309, 4447107.109592, 4446643.796876, ..., 3337009.840791, 3336546.528075, 3336083.215358])
- x(x)float64-1.001e+07 ... -8.896e+06
array([-10007323.020642, -10006859.707925, -10006396.395209, ..., -8896762.439124, -8896299.126408, -8895835.813691])
- band(band)int641
array([1])
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -10007554.677 463.3127165279165 0.0 4447802.078667 0.0 -463.3127165279167
array(0)
- num_observations_500m(band, y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- Number of Observations
- units :
- none
[5760000 values with dtype=float32]
- sur_refl_b01_1(band, y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 1 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b02_1(band, y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 2 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b03_1(band, y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 3 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b04_1(band, y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 4 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b05_1(band, y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 5 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b06_1(band, y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 6 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b07_1(band, y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 7 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- QC_500m_1(band, y, x)float64...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- 500m Reflectance Band Quality - first layer
- units :
- bit field
[5760000 values with dtype=float64]
- obscov_500m_1(band, y, x)float32...
- scale_factor :
- 0.00999999977648258
- add_offset :
- 0.0
- long_name :
- Observation coverage - first layer
- units :
- percent
[5760000 values with dtype=float32]
- iobs_res_1(band, y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- observation number in coarser grid - first layer
- units :
- none
[5760000 values with dtype=float32]
- q_scan_1(band, y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- 250m scan value information - first layer
- units :
- none
[5760000 values with dtype=float32]
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
Subset Data By Group or Variable
If you need to open the entire dataset, you can follow the steps above. Alternatively you can specific subgroups or even layers / variables
in the data to open specifically using the group=
parameter.
There are a few ways to get the group names. One manual way is to use the HDF4 tool (or something like panoply) to view the
groups. You could also use something like gdalinfo
or rasterio to loop through groups and subgroups.
The files with this pattern in the name:
sur_refl_b01_1
are the bands which contain surface reflectance data.
- sur_refl_b01_1: MODIS Band One
- sur_refl_b02_1: MODIS Band Two
etc.
Notice that there are some other layers in the file as well including the state_1km
layer which contains the QA (cloud and quality assurance) information.
# Use rasterio to print all of the subdataset names in the data
# Here you can see the group names: MODIS_Grid_500m_2D & MODIS_Grid_1km_2D
import rasterio as rio
with rio.open(modis_pre_path) as groups:
for name in groups.subdatasets:
print(name)
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:num_observations_1km
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:granule_pnt_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:num_observations_500m
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:sur_refl_b01_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:sur_refl_b02_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:sur_refl_b03_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:sur_refl_b04_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:sur_refl_b05_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:sur_refl_b06_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:sur_refl_b07_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:QC_500m_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:state_1km_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:obscov_500m_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:iobs_res_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_500m_2D:q_scan_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:SensorZenith_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:SensorAzimuth_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:Range_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:SolarZenith_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:SolarAzimuth_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:gflags_1
HDF4_EOS:EOS_GRID:cold-springs-modis-h4/07_july_2016/MOD09GA.A2016189.h09v05.006.2016191073856.hdf:MODIS_Grid_1km_2D:orbit_pnt_1
Below you actually open the data subsetting first by
- group and then
- by variable names
# Subset by group only - Notice you have all bands in the returned object
rxr.open_rasterio(modis_pre_path,
masked=True,
group="MODIS_Grid_500m_2D").squeeze()
<xarray.Dataset> Dimensions: (y: 2400, x: 2400) Coordinates: * y (y) float64 4.448e+06 4.447e+06 ... 3.336e+06 * x (x) float64 -1.001e+07 -1.001e+07 ... -8.896e+06 band int64 1 spatial_ref int64 0 Data variables: num_observations_500m (y, x) float32 ... sur_refl_b01_1 (y, x) float32 ... sur_refl_b02_1 (y, x) float32 ... sur_refl_b03_1 (y, x) float32 ... sur_refl_b04_1 (y, x) float32 ... sur_refl_b05_1 (y, x) float32 ... sur_refl_b06_1 (y, x) float32 ... sur_refl_b07_1 (y, x) float32 ... QC_500m_1 (y, x) float64 ... obscov_500m_1 (y, x) float32 ... iobs_res_1 (y, x) float32 ... q_scan_1 (y, x) float32 ... Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- y: 2400
- x: 2400
- y(y)float644.448e+06 4.447e+06 ... 3.336e+06
array([4447570.422309, 4447107.109592, 4446643.796876, ..., 3337009.840791, 3336546.528075, 3336083.215358])
- x(x)float64-1.001e+07 ... -8.896e+06
array([-10007323.020642, -10006859.707925, -10006396.395209, ..., -8896762.439124, -8896299.126408, -8895835.813691])
- band()int641
array(1)
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -10007554.677 463.3127165279165 0.0 4447802.078667 0.0 -463.3127165279167
array(0)
- num_observations_500m(y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- Number of Observations
- units :
- none
[5760000 values with dtype=float32]
- sur_refl_b01_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 1 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b02_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 2 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b03_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 3 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b04_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 4 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b05_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 5 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b06_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 6 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b07_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 7 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- QC_500m_1(y, x)float64...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- 500m Reflectance Band Quality - first layer
- units :
- bit field
[5760000 values with dtype=float64]
- obscov_500m_1(y, x)float32...
- scale_factor :
- 0.00999999977648258
- add_offset :
- 0.0
- long_name :
- Observation coverage - first layer
- units :
- percent
[5760000 values with dtype=float32]
- iobs_res_1(y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- observation number in coarser grid - first layer
- units :
- none
[5760000 values with dtype=float32]
- q_scan_1(y, x)float32...
- scale_factor :
- 1.0
- add_offset :
- 0.0
- long_name :
- 250m scan value information - first layer
- units :
- none
[5760000 values with dtype=float32]
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
Subset by a list of variable names.
# Open just the bands that you want to process
desired_bands = ["sur_refl_b01_1",
"sur_refl_b02_1",
"sur_refl_b03_1",
"sur_refl_b04_1",
"sur_refl_b07_1"]
# Notice that here, you get a single xarray object with just the bands that
# you want to work with
modis_pre_bands = rxr.open_rasterio(modis_pre_path,
variable=desired_bands).squeeze()
modis_pre_bands
<xarray.Dataset> Dimensions: (y: 2400, x: 2400) Coordinates: * y (y) float64 4.448e+06 4.447e+06 ... 3.337e+06 3.336e+06 * x (x) float64 -1.001e+07 -1.001e+07 ... -8.896e+06 -8.896e+06 band int64 1 spatial_ref int64 0 Data variables: sur_refl_b01_1 (y, x) int16 ... sur_refl_b02_1 (y, x) int16 ... sur_refl_b03_1 (y, x) int16 ... sur_refl_b04_1 (y, x) int16 ... sur_refl_b07_1 (y, x) int16 ... Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- y: 2400
- x: 2400
- y(y)float644.448e+06 4.447e+06 ... 3.336e+06
array([4447570.422309, 4447107.109592, 4446643.796876, ..., 3337009.840791, 3336546.528075, 3336083.215358])
- x(x)float64-1.001e+07 ... -8.896e+06
array([-10007323.020642, -10006859.707925, -10006396.395209, ..., -8896762.439124, -8896299.126408, -8895835.813691])
- band()int641
array(1)
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -10007554.677 463.3127165279165 0.0 4447802.078667 0.0 -463.3127165279167
array(0)
- sur_refl_b01_1(y, x)int16...
- _FillValue :
- -28672.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 1 - first layer
- units :
- reflectance
[5760000 values with dtype=int16]
- sur_refl_b02_1(y, x)int16...
- _FillValue :
- -28672.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 2 - first layer
- units :
- reflectance
[5760000 values with dtype=int16]
- sur_refl_b03_1(y, x)int16...
- _FillValue :
- -28672.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 3 - first layer
- units :
- reflectance
[5760000 values with dtype=int16]
- sur_refl_b04_1(y, x)int16...
- _FillValue :
- -28672.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 4 - first layer
- units :
- reflectance
[5760000 values with dtype=int16]
- sur_refl_b07_1(y, x)int16...
- _FillValue :
- -28672.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 7 - first layer
- units :
- reflectance
[5760000 values with dtype=int16]
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
# view nodata value
modis_pre_bands.sur_refl_b01_1.rio.nodata
-28672
Handle NoData Using Masked=True
If you look at the MODIS documentation table that is in the Introduction to MODIS chapter, you will see that the range of value values for MODIS spans from -100 to 16000. There is also a fill or no data value -28672 to consider. By using the masked=True
parameter when you open the data, you mask all pixels that are nodata
.
Below you open the data and mask no data values using masked=True
. Notice that once you apply the mask, the nodata
value
attribute is erased from the object.
# Open just the bands that you want to process
desired_bands = ["sur_refl_b01_1",
"sur_refl_b02_1",
"sur_refl_b03_1",
"sur_refl_b04_1",
"sur_refl_b07_1"]
# Notice that here, you get a single xarray object with just the bands that
# you want to work with
modis_pre_bands = rxr.open_rasterio(modis_pre_path,
masked=True,
variable=desired_bands).squeeze()
modis_pre_bands
<xarray.Dataset> Dimensions: (y: 2400, x: 2400) Coordinates: * y (y) float64 4.448e+06 4.447e+06 ... 3.337e+06 3.336e+06 * x (x) float64 -1.001e+07 -1.001e+07 ... -8.896e+06 -8.896e+06 band int64 1 spatial_ref int64 0 Data variables: sur_refl_b01_1 (y, x) float32 ... sur_refl_b02_1 (y, x) float32 ... sur_refl_b03_1 (y, x) float32 ... sur_refl_b04_1 (y, x) float32 ... sur_refl_b07_1 (y, x) float32 ... Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- y: 2400
- x: 2400
- y(y)float644.448e+06 4.447e+06 ... 3.336e+06
array([4447570.422309, 4447107.109592, 4446643.796876, ..., 3337009.840791, 3336546.528075, 3336083.215358])
- x(x)float64-1.001e+07 ... -8.896e+06
array([-10007323.020642, -10006859.707925, -10006396.395209, ..., -8896762.439124, -8896299.126408, -8895835.813691])
- band()int641
array(1)
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -10007554.677 463.3127165279165 0.0 4447802.078667 0.0 -463.3127165279167
array(0)
- sur_refl_b01_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 1 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b02_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 2 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b03_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 3 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b04_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 4 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- sur_refl_b07_1(y, x)float32...
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 7 - first layer
- units :
- reflectance
[5760000 values with dtype=float32]
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
# Now your nodata value returns a NAN as it has been masked
# and accounted for
modis_pre_bands.sur_refl_b01_1.rio.nodata
nan
Process MODIS Bands Stored in a HDF4 File
Xarray has several built in plot methods making it easy to explore your data. Below you plot the first band.
# Plot band one of the data
ep.plot_bands(modis_pre_bands.sur_refl_b01_1)
plt.show()
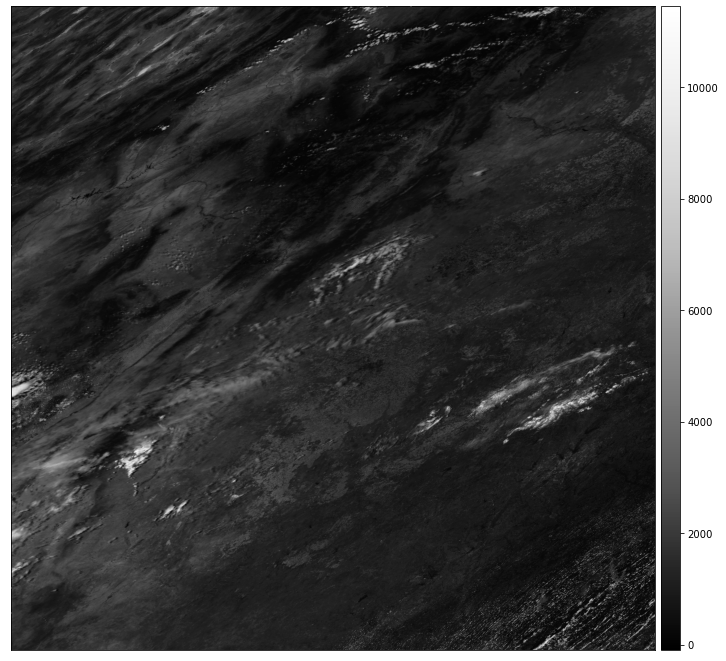
# Note that you can also call the data variable by name
ep.plot_bands(modis_pre_bands["sur_refl_b01_1"])
plt.show()
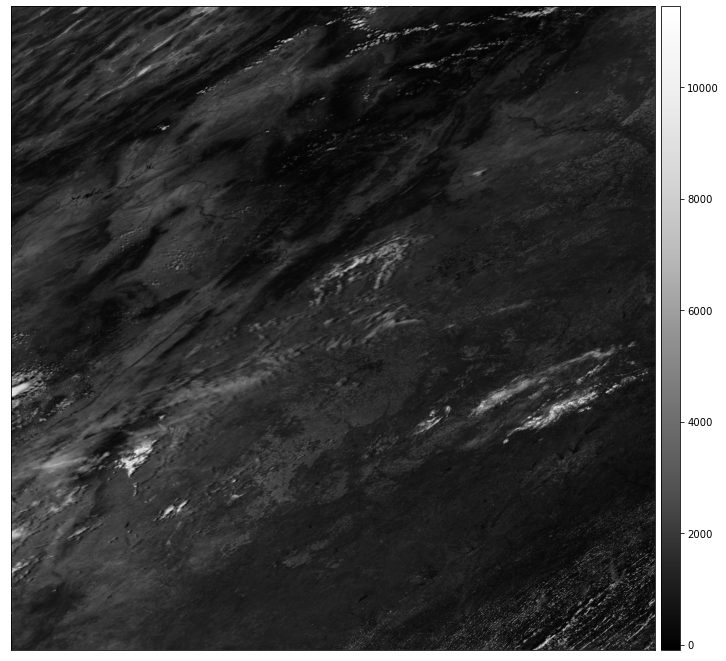
Plot All MODIS Bands with EarthPy
Now that you have the needed reflectance data, you can plot your data using earthpy.plot_bands.
To plot all bands in the data, you will need to first create an Xarray DataArray object.
print(type(modis_pre_bands))
print(type(modis_pre_bands.to_array()))
# You can plot each band easily using a data array object
modis_pre_bands.to_array()
<class 'xarray.core.dataset.Dataset'>
<class 'xarray.core.dataarray.DataArray'>
<xarray.DataArray (variable: 5, y: 2400, x: 2400)> array([[[1902., 1949., 1947., ..., 1327., 1327., 1181.], [1949., 2160., 2095., ..., 1327., 1273., 1273.], [2054., 2156., 2187., ..., 1139., 1101., 1206.], ..., [1387., 1469., 1469., ..., 343., 499., 1006.], [1298., 1316., 1469., ..., 905., 499., 436.], [1316., 1316., 1454., ..., 905., 578., 351.]], [[2714., 2859., 3041., ..., 3046., 3046., 4421.], [2968., 3362., 3253., ..., 3046., 4550., 4550.], [3198., 3315., 3246., ..., 4178., 4017., 3992.], ..., [2911., 2947., 2947., ..., 4059., 4907., 3799.], [2954., 2890., 2947., ..., 4517., 4907., 3459.], [2890., 2890., 2935., ..., 4517., 3728., 4021.]], [[1056., 1072., 1012., ..., 745., 745., 581.], [1051., 1150., 1039., ..., 745., 595., 595.], [1073., 1220., 1152., ..., 588., 564., 563.], ..., [ 759., 813., 813., ..., 150., 218., 852.], [ 703., 735., 813., ..., 585., 218., 199.], [ 735., 735., 798., ..., 585., 338., 109.]], [[1534., 1567., 1527., ..., 1107., 1107., 1069.], [1548., 1776., 1665., ..., 1107., 1121., 1121.], [1696., 1813., 1820., ..., 1029., 1018., 1077.], ..., [1209., 1267., 1267., ..., 597., 788., 1153.], [1165., 1154., 1267., ..., 1089., 788., 603.], [1154., 1154., 1231., ..., 1089., 759., 516.]], [[2781., 2692., 2801., ..., 2260., 2260., 1534.], [2792., 2705., 2684., ..., 2260., 1449., 1449.], [2747., 2754., 2733., ..., 1713., 1802., 1548.], ..., [2457., 2446., 2446., ..., 444., 832., 1379.], [2289., 2296., 2446., ..., 1161., 832., 762.], [2296., 2296., 2511., ..., 1161., 913., 703.]]], dtype=float32) Coordinates: * y (y) float64 4.448e+06 4.447e+06 ... 3.337e+06 3.336e+06 * x (x) float64 -1.001e+07 -1.001e+07 ... -8.896e+06 -8.896e+06 band int64 1 spatial_ref int64 0 * variable (variable) <U14 'sur_refl_b01_1' ... 'sur_refl_b07_1' Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- variable: 5
- y: 2400
- x: 2400
- 1.902e+03 1.949e+03 1.947e+03 2.095e+03 ... 1.161e+03 913.0 703.0
array([[[1902., 1949., 1947., ..., 1327., 1327., 1181.], [1949., 2160., 2095., ..., 1327., 1273., 1273.], [2054., 2156., 2187., ..., 1139., 1101., 1206.], ..., [1387., 1469., 1469., ..., 343., 499., 1006.], [1298., 1316., 1469., ..., 905., 499., 436.], [1316., 1316., 1454., ..., 905., 578., 351.]], [[2714., 2859., 3041., ..., 3046., 3046., 4421.], [2968., 3362., 3253., ..., 3046., 4550., 4550.], [3198., 3315., 3246., ..., 4178., 4017., 3992.], ..., [2911., 2947., 2947., ..., 4059., 4907., 3799.], [2954., 2890., 2947., ..., 4517., 4907., 3459.], [2890., 2890., 2935., ..., 4517., 3728., 4021.]], [[1056., 1072., 1012., ..., 745., 745., 581.], [1051., 1150., 1039., ..., 745., 595., 595.], [1073., 1220., 1152., ..., 588., 564., 563.], ..., [ 759., 813., 813., ..., 150., 218., 852.], [ 703., 735., 813., ..., 585., 218., 199.], [ 735., 735., 798., ..., 585., 338., 109.]], [[1534., 1567., 1527., ..., 1107., 1107., 1069.], [1548., 1776., 1665., ..., 1107., 1121., 1121.], [1696., 1813., 1820., ..., 1029., 1018., 1077.], ..., [1209., 1267., 1267., ..., 597., 788., 1153.], [1165., 1154., 1267., ..., 1089., 788., 603.], [1154., 1154., 1231., ..., 1089., 759., 516.]], [[2781., 2692., 2801., ..., 2260., 2260., 1534.], [2792., 2705., 2684., ..., 2260., 1449., 1449.], [2747., 2754., 2733., ..., 1713., 1802., 1548.], ..., [2457., 2446., 2446., ..., 444., 832., 1379.], [2289., 2296., 2446., ..., 1161., 832., 762.], [2296., 2296., 2511., ..., 1161., 913., 703.]]], dtype=float32)
- y(y)float644.448e+06 4.447e+06 ... 3.336e+06
array([4447570.422309, 4447107.109592, 4446643.796876, ..., 3337009.840791, 3336546.528075, 3336083.215358])
- x(x)float64-1.001e+07 ... -8.896e+06
array([-10007323.020642, -10006859.707925, -10006396.395209, ..., -8896762.439124, -8896299.126408, -8895835.813691])
- band()int641
array(1)
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -10007554.677 463.3127165279165 0.0 4447802.078667 0.0 -463.3127165279167
array(0)
- variable(variable)<U14'sur_refl_b01_1' ... 'sur_refl_b...
array(['sur_refl_b01_1', 'sur_refl_b02_1', 'sur_refl_b03_1', 'sur_refl_b04_1', 'sur_refl_b07_1'], dtype='<U14')
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
# Plot the data as a DataArray
# This is only a data exploration step
ep.plot_bands(modis_pre_bands.to_array().values,
figsize=(10, 6))
plt.show()
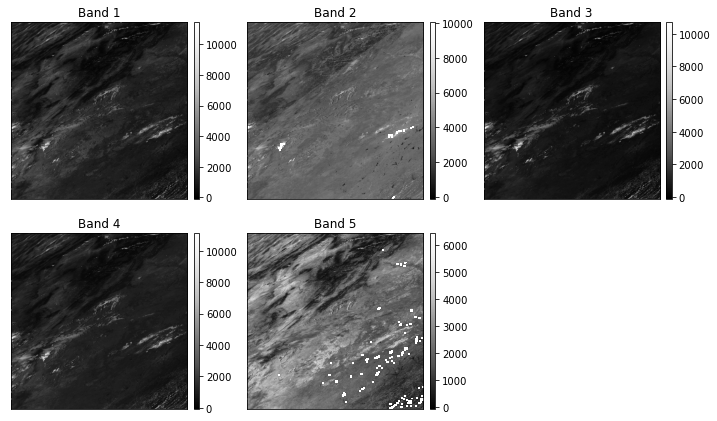
RGB Image of MODIS Data Using EarthPy
Once you have cleaned your data cleaned, you can plot an RGB image using earthpy.
# Select the rgb bands only
rgb_bands = ['sur_refl_b01_1',
'sur_refl_b03_1',
'sur_refl_b04_1']
# Turn the data into a DataArray
modis_rgb_xr = modis_pre_bands[rgb_bands].to_array()
modis_rgb_xr
<xarray.DataArray (variable: 3, y: 2400, x: 2400)> array([[[1902., 1949., 1947., ..., 1327., 1327., 1181.], [1949., 2160., 2095., ..., 1327., 1273., 1273.], [2054., 2156., 2187., ..., 1139., 1101., 1206.], ..., [1387., 1469., 1469., ..., 343., 499., 1006.], [1298., 1316., 1469., ..., 905., 499., 436.], [1316., 1316., 1454., ..., 905., 578., 351.]], [[1056., 1072., 1012., ..., 745., 745., 581.], [1051., 1150., 1039., ..., 745., 595., 595.], [1073., 1220., 1152., ..., 588., 564., 563.], ..., [ 759., 813., 813., ..., 150., 218., 852.], [ 703., 735., 813., ..., 585., 218., 199.], [ 735., 735., 798., ..., 585., 338., 109.]], [[1534., 1567., 1527., ..., 1107., 1107., 1069.], [1548., 1776., 1665., ..., 1107., 1121., 1121.], [1696., 1813., 1820., ..., 1029., 1018., 1077.], ..., [1209., 1267., 1267., ..., 597., 788., 1153.], [1165., 1154., 1267., ..., 1089., 788., 603.], [1154., 1154., 1231., ..., 1089., 759., 516.]]], dtype=float32) Coordinates: * y (y) float64 4.448e+06 4.447e+06 ... 3.337e+06 3.336e+06 * x (x) float64 -1.001e+07 -1.001e+07 ... -8.896e+06 -8.896e+06 band int64 1 spatial_ref int64 0 * variable (variable) <U14 'sur_refl_b01_1' ... 'sur_refl_b04_1' Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- variable: 3
- y: 2400
- x: 2400
- 1.902e+03 1.949e+03 1.947e+03 2.095e+03 ... 1.089e+03 759.0 516.0
array([[[1902., 1949., 1947., ..., 1327., 1327., 1181.], [1949., 2160., 2095., ..., 1327., 1273., 1273.], [2054., 2156., 2187., ..., 1139., 1101., 1206.], ..., [1387., 1469., 1469., ..., 343., 499., 1006.], [1298., 1316., 1469., ..., 905., 499., 436.], [1316., 1316., 1454., ..., 905., 578., 351.]], [[1056., 1072., 1012., ..., 745., 745., 581.], [1051., 1150., 1039., ..., 745., 595., 595.], [1073., 1220., 1152., ..., 588., 564., 563.], ..., [ 759., 813., 813., ..., 150., 218., 852.], [ 703., 735., 813., ..., 585., 218., 199.], [ 735., 735., 798., ..., 585., 338., 109.]], [[1534., 1567., 1527., ..., 1107., 1107., 1069.], [1548., 1776., 1665., ..., 1107., 1121., 1121.], [1696., 1813., 1820., ..., 1029., 1018., 1077.], ..., [1209., 1267., 1267., ..., 597., 788., 1153.], [1165., 1154., 1267., ..., 1089., 788., 603.], [1154., 1154., 1231., ..., 1089., 759., 516.]]], dtype=float32)
- y(y)float644.448e+06 4.447e+06 ... 3.336e+06
array([4447570.422309, 4447107.109592, 4446643.796876, ..., 3337009.840791, 3336546.528075, 3336083.215358])
- x(x)float64-1.001e+07 ... -8.896e+06
array([-10007323.020642, -10006859.707925, -10006396.395209, ..., -8896762.439124, -8896299.126408, -8895835.813691])
- band()int641
array(1)
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -10007554.677 463.3127165279165 0.0 4447802.078667 0.0 -463.3127165279167
array(0)
- variable(variable)<U14'sur_refl_b01_1' ... 'sur_refl_b...
array(['sur_refl_b01_1', 'sur_refl_b03_1', 'sur_refl_b04_1'], dtype='<U14')
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
# Plot MODIS RGB numpy image array
ep.plot_rgb(modis_rgb_xr.values,
rgb=[0, 2, 1],
title='RGB Image of MODIS Data')
plt.show()
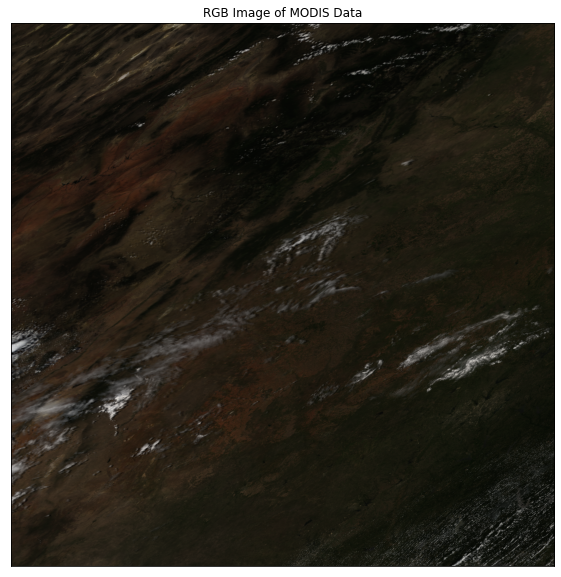
Crop (Clip) MODIS Data Using Rioxarray
Above you opened and plotted MODIS reflectance data. However, the data cover a larger study area than you need. It is a good idea to crop your the data to maximize processing efficiency. Less pixels means less
processing time and power needed.
There are a few approaches here to consider
- Do you want to clip the data to a box (a rectangular extent) vs to the specific geometry of your shapefile
- If your data are large and you have limited memory, you may only want to open the data needed for processing.
Below you have a few different options for clipping your data. To demonstrate the full workflow, we will s tart over and open the data.
To begin, open up the fire boundary which contains your study area extent that you wish to crop your MODIS data to co_cold_springs_20160711_2200_dd83.shp
).
# Open fire boundary
fire_boundary_path = os.path.join("cold-springs-fire",
"vector_layers",
"fire-boundary-geomac",
"co_cold_springs_20160711_2200_dd83.shp")
fire_boundary = gpd.read_file(fire_boundary_path)
# Check the CRS of your study area extent
fire_boundary.crs
<Geographic 2D CRS: EPSG:4269>
Name: NAD83
Axis Info [ellipsoidal]:
- Lat[north]: Geodetic latitude (degree)
- Lon[east]: Geodetic longitude (degree)
Area of Use:
- name: North America - onshore and offshore: Canada - Alberta; British Columbia; Manitoba; New Brunswick; Newfoundland and Labrador; Northwest Territories; Nova Scotia; Nunavut; Ontario; Prince Edward Island; Quebec; Saskatchewan; Yukon. Puerto Rico. United States (USA) - Alabama; Alaska; Arizona; Arkansas; California; Colorado; Connecticut; Delaware; Florida; Georgia; Hawaii; Idaho; Illinois; Indiana; Iowa; Kansas; Kentucky; Louisiana; Maine; Maryland; Massachusetts; Michigan; Minnesota; Mississippi; Missouri; Montana; Nebraska; Nevada; New Hampshire; New Jersey; New Mexico; New York; North Carolina; North Dakota; Ohio; Oklahoma; Oregon; Pennsylvania; Rhode Island; South Carolina; South Dakota; Tennessee; Texas; Utah; Vermont; Virginia; Washington; West Virginia; Wisconsin; Wyoming. US Virgin Islands. British Virgin Islands.
- bounds: (167.65, 14.92, -47.74, 86.46)
Datum: North American Datum 1983
- Ellipsoid: GRS 1980
- Prime Meridian: Greenwich
Reproject the Clip Extent (Fire Boundary)
To check the CRS of MODIS data, you can:
- Open the MODIS data in
rioxarray
- Grab the CRS of the band accessing
rio.crs
from therioxarray
object.
You can also use rasterio to “view” and access the CRS
You can then use the CRS of the band to check and reproject the fire boundary if it is not in the same CRS.
In the code below, you use these steps to reproject the fire boundary to match the HDF4 data.
Note that you do not need all of the print statments included below. They are included to help you see what is happening in the code!
# Check CRS
if not fire_boundary.crs == modis_rgb_xr.rio.crs:
# If the crs is not equal reproject the data
fire_bound_sin = fire_boundary.to_crs(modis_rgb_xr.rio.crs)
fire_bound_sin.crs
<Projected CRS: PROJCS["unnamed",GEOGCS["Unknown datum based upon ...>
Name: unnamed
Axis Info [cartesian]:
- [east]: Easting (Meter)
- [north]: Northing (Meter)
Area of Use:
- undefined
Coordinate Operation:
- name: unnamed
- method: Sinusoidal
Datum: Not specified (based on custom spheroid)
- Ellipsoid: Custom spheroid
- Prime Meridian: Greenwich
Scenario 1: Open and Clip the Data Using the CRS=Parameter
In the first scenario obelow, you open and clip your data. By using the crs=
parameter, you can skip needing to reproject the clip extent as rioxarray does it for you.
The caveat too this approach is that it is generally slower than the other approaches. The benefit is it avoids the additional reprojection step.
# Notice this is a box - representing the spatial extent
# of your study area
crop_bound_box = [box(*fire_boundary.total_bounds)]
crop_bound_box[0]
# Notice that this is the actual shape of the fire boundary
# First you need to decide whether you want to clip to the
# Box / extent (above) or the shape that you see here.
fire_boundary.geometry[0]
Below you clip the data to the box extent.
A few notes on each parameter included below
open_rasterio
parameters:
masked=True
: This masks out no data values for youvariable=
: Allows you to select data variables to open effectively subsetting or slicing out only the parts of the data that you need.
rio.clip
parameters
crs=
: Use this if your crop extent is in a different CRS than your raster xarray data. NOTE that this can slow your processing down significantly.all_touched=
: Set to True if you want to include all pixels touched by your crop boundary. Sometimes the edge of a shapefile will cross over a pixel making it a “partial” pixel. Do you want to include all pixels even if only some of that pixel is in your study area? IF so set to True. If you only with to include pixels that are FULLY WITHIN your study area set toFalse
.from_disk
: Include this to only OPEN the data that you wish to work with. Thus instead of opening up the entire raster and then clipping, the data within the clip boundary are “sliced” out. this saves memory and sometimes processing time!
# Open just the bands that you want to process
desired_bands = ["sur_refl_b01_1",
"sur_refl_b02_1",
"sur_refl_b03_1",
"sur_refl_b04_1",
"sur_refl_b07_1"]
# Create a box representing the spatial extent of your data
crop_bound_box = [box(*fire_boundary.total_bounds)]
# Clip the data by chaining together rio.clip with rio.open_rasterio
# from_disk=True allows you to only open the data that you wish to work with
modis_pre_clip = rxr.open_rasterio(modis_pre_path,
masked=True,
variable=desired_bands).rio.clip(crop_bound_box,
crs=fire_boundary.crs,
# Include all pixels even partial pixels
all_touched=True,
from_disk=True).squeeze()
# The final clipped data
modis_pre_clip
<xarray.Dataset> Dimensions: (y: 3, x: 12) Coordinates: * y (y) float64 4.446e+06 4.446e+06 4.445e+06 * x (x) float64 -8.989e+06 -8.989e+06 ... -8.985e+06 -8.984e+06 band int64 1 spatial_ref int64 0 Data variables: sur_refl_b01_1 (y, x) float32 nan nan 428.0 450.0 ... 458.0 nan nan nan sur_refl_b02_1 (y, x) float32 nan nan 3.013e+03 2.809e+03 ... nan nan nan sur_refl_b03_1 (y, x) float32 nan nan 259.0 235.0 ... 265.0 nan nan nan sur_refl_b04_1 (y, x) float32 nan nan 563.0 541.0 ... 518.0 nan nan nan sur_refl_b07_1 (y, x) float32 nan nan 832.0 820.0 ... 804.0 nan nan nan Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- y: 3
- x: 12
- y(y)float644.446e+06 4.446e+06 4.445e+06
- axis :
- Y
- long_name :
- y coordinate of projection
- standard_name :
- projection_y_coordinate
- units :
- metre
array([4446180.484159, 4445717.171443, 4445253.858726])
- x(x)float64-8.989e+06 ... -8.984e+06
- axis :
- X
- long_name :
- x coordinate of projection
- standard_name :
- projection_x_coordinate
- units :
- metre
array([-8989424.98243 , -8988961.669713, -8988498.356997, -8988035.04428 , -8987571.731564, -8987108.418847, -8986645.106131, -8986181.793414, -8985718.480698, -8985255.167981, -8984791.855265, -8984328.542548])
- band()int641
array(1)
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -8989656.638788167 463.3127165279266 0.0 4446412.1405174155 0.0 -463.312716527842
array(0)
- sur_refl_b01_1(y, x)float32nan nan 428.0 450.0 ... nan nan nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 1 - first layer
- units :
- reflectance
array([[ nan, nan, 428., 450., 450., 438., 438., 421., 421., 409., 409., 483.], [ nan, 402., 402., 402., 402., 442., 442., 442., 442., 458., 458., nan], [402., 402., 402., 402., 442., 442., 442., 442., 458., nan, nan, nan]], dtype=float32)
- sur_refl_b02_1(y, x)float32nan nan 3.013e+03 ... nan nan nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 2 - first layer
- units :
- reflectance
array([[ nan, nan, 3013., 2809., 2809., 2730., 2730., 2628., 2628., 2641., 2641., 2576.], [ nan, 2565., 2565., 2565., 2565., 2522., 2522., 2522., 2522., 2496., 2496., nan], [2565., 2565., 2565., 2565., 2522., 2522., 2522., 2522., 2496., nan, nan, nan]], dtype=float32)
- sur_refl_b03_1(y, x)float32nan nan 259.0 235.0 ... nan nan nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 3 - first layer
- units :
- reflectance
array([[ nan, nan, 259., 235., 235., 231., 231., 211., 211., 203., 203., 245.], [ nan, 217., 217., 217., 217., 230., 230., 230., 230., 265., 265., nan], [217., 217., 217., 217., 230., 230., 230., 230., 265., nan, nan, nan]], dtype=float32)
- sur_refl_b04_1(y, x)float32nan nan 563.0 541.0 ... nan nan nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 4 - first layer
- units :
- reflectance
array([[ nan, nan, 563., 541., 541., 528., 528., 494., 494., 486., 486., 530.], [ nan, 476., 476., 476., 476., 476., 476., 476., 476., 518., 518., nan], [476., 476., 476., 476., 476., 476., 476., 476., 518., nan, nan, nan]], dtype=float32)
- sur_refl_b07_1(y, x)float32nan nan 832.0 820.0 ... nan nan nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 7 - first layer
- units :
- reflectance
array([[ nan, nan, 832., 820., 820., 820., 820., 749., 749., 715., 715., 820.], [ nan, 715., 715., 715., 715., 789., 789., 789., 789., 804., 804., nan], [715., 715., 715., 715., 789., 789., 789., 789., 804., nan, nan, nan]], dtype=float32)
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
# For demonstration purpposes i'm creating a plotting extent
from rasterio.plot import plotting_extent
modis_ext = plotting_extent(modis_pre_clip.to_array().values[0],
modis_pre_clip.rio.transform())
# View cropped data
f, ax = plt.subplots()
ep.plot_bands(modis_pre_clip.to_array().values[0],
ax=ax,
extent=modis_ext,
title="Plot of data clipped to the crop box (extent)")
fire_bound_sin.plot(ax=ax,
color="green")
plt.show()
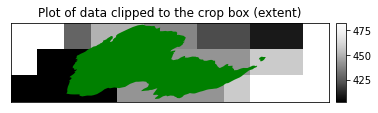
Scenario 2: Crop Using the GEOMETRY
In the scenario below you crop / mask the data to the actual geometry shape of the fire boundary. Because the MODIS pixels are larger and the study area is relative small, you can’t visually see a difference however you will see a difference with landsat and other higher resolution data.
# Open just the bands that you want to process
desired_bands = ["sur_refl_b01_1",
"sur_refl_b02_1",
"sur_refl_b03_1",
"sur_refl_b04_1",
"sur_refl_b07_1"]
# Create a box representing the spatial extent of your data
crop_bound_box = [box(*fire_boundary.total_bounds)]
# Clip the data by chaining together rio.clip with rio.open_rasterio
# from_disk=True allows you to only open the data that you wish to work with
modis_pre_clip_geom = rxr.open_rasterio(modis_pre_path,
masked=True,
variable=desired_bands).rio.clip(fire_boundary.geometry.apply(mapping),
crs=fire_boundary.crs,
# Include all pixels even partial pixels
all_touched=True,
from_disk=True).squeeze()
# The final clipped data - Notice there are fewer pixels
# In the output object
modis_pre_clip_geom
<xarray.Dataset> Dimensions: (y: 3, x: 8) Coordinates: * y (y) float64 4.446e+06 4.446e+06 4.445e+06 * x (x) float64 -8.988e+06 -8.988e+06 ... -8.986e+06 -8.985e+06 band int64 1 spatial_ref int64 0 Data variables: sur_refl_b01_1 (y, x) float32 428.0 450.0 450.0 438.0 ... 442.0 458.0 nan sur_refl_b02_1 (y, x) float32 3.013e+03 2.809e+03 ... 2.496e+03 nan sur_refl_b03_1 (y, x) float32 259.0 235.0 235.0 231.0 ... 230.0 265.0 nan sur_refl_b04_1 (y, x) float32 563.0 541.0 541.0 528.0 ... 476.0 518.0 nan sur_refl_b07_1 (y, x) float32 832.0 820.0 820.0 820.0 ... 789.0 804.0 nan Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- y: 3
- x: 8
- y(y)float644.446e+06 4.446e+06 4.445e+06
- axis :
- Y
- long_name :
- y coordinate of projection
- standard_name :
- projection_y_coordinate
- units :
- metre
array([4446180.484159, 4445717.171443, 4445253.858726])
- x(x)float64-8.988e+06 ... -8.985e+06
- axis :
- X
- long_name :
- x coordinate of projection
- standard_name :
- projection_x_coordinate
- units :
- metre
array([-8988498.356997, -8988035.04428 , -8987571.731564, -8987108.418847, -8986645.106131, -8986181.793414, -8985718.480698, -8985255.167981])
- band()int641
array(1)
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -8988730.01335511 463.3127165277089 0.0 4446412.1405174155 0.0 -463.312716527842
array(0)
- sur_refl_b01_1(y, x)float32428.0 450.0 450.0 ... 458.0 nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 1 - first layer
- units :
- reflectance
array([[428., 450., 450., 438., 438., nan, nan, nan], [402., 402., 402., 442., 442., 442., 442., 458.], [402., 402., 442., 442., 442., 442., 458., nan]], dtype=float32)
- sur_refl_b02_1(y, x)float323.013e+03 2.809e+03 ... nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 2 - first layer
- units :
- reflectance
array([[3013., 2809., 2809., 2730., 2730., nan, nan, nan], [2565., 2565., 2565., 2522., 2522., 2522., 2522., 2496.], [2565., 2565., 2522., 2522., 2522., 2522., 2496., nan]], dtype=float32)
- sur_refl_b03_1(y, x)float32259.0 235.0 235.0 ... 265.0 nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 3 - first layer
- units :
- reflectance
array([[259., 235., 235., 231., 231., nan, nan, nan], [217., 217., 217., 230., 230., 230., 230., 265.], [217., 217., 230., 230., 230., 230., 265., nan]], dtype=float32)
- sur_refl_b04_1(y, x)float32563.0 541.0 541.0 ... 518.0 nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 4 - first layer
- units :
- reflectance
array([[563., 541., 541., 528., 528., nan, nan, nan], [476., 476., 476., 476., 476., 476., 476., 518.], [476., 476., 476., 476., 476., 476., 518., nan]], dtype=float32)
- sur_refl_b07_1(y, x)float32832.0 820.0 820.0 ... 804.0 nan
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 7 - first layer
- units :
- reflectance
array([[832., 820., 820., 820., 820., nan, nan, nan], [715., 715., 715., 789., 789., 789., 789., 804.], [715., 715., 789., 789., 789., 789., 804., nan]], dtype=float32)
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
Note that the difference between the two plots will be more visible when using data like landsat with smaller pixels.
# View cropped data - note the different b etew
f, ax = plt.subplots()
ep.plot_bands(modis_pre_clip_geom.to_array().values[0],
ax=ax,
extent=modis_ext,
title="Plot of the data clipped to the geometry")
fire_bound_sin.plot(ax=ax,
color="green")
plt.show()
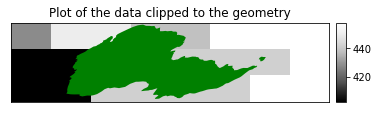
Scenario 3: Crop MODIS Data Using Reprojected Fire Boundary
In the example below, you skip the crs=
argument.
This has assumed that you have already reprojected your
vector crop extent.
You can get the crs without opening up the whole dataset using the function es.crs_check
from the EarthPy package. If you were to open the dataset wihout clipping it, it’s less effecient. More data would be stored in memory in such a case. Which is why we use es.crs_check
to get the crs without opening up the data fully.
Once you have opened and reprojected your crop extent, you can open and crop your MODIS raster data. You can do this like you did above, just be sure to leave out the crs=
argument, since the data has already been reprojected! To clip to the box, call .clip([box(*boundary_name.total_bounds)])
. Keep in mind, for this to work you have to import box
from shapely.geometry
!
# Get the crs from the dataset with es.crs_check
modis_bands_crs = es.crs_check(modis_pre_path)
# Reproject the fire boundary
fire_boundary_sin = fire_boundary.to_crs(modis_bands_crs)
# Open and crop the data with the box made from the reprojected fire boundary
modis_bands_clip = rxr.open_rasterio(modis_pre_path,
masked=True,
variable=desired_bands).rio.clip(
[box(*fire_bound_sin.total_bounds)],
all_touched=True,
from_disk=True).squeeze()
modis_bands_clip
<xarray.Dataset> Dimensions: (y: 3, x: 8) Coordinates: * y (y) float64 4.446e+06 4.446e+06 4.445e+06 * x (x) float64 -8.988e+06 -8.988e+06 ... -8.986e+06 -8.985e+06 band int64 1 spatial_ref int64 0 Data variables: sur_refl_b01_1 (y, x) float32 428.0 450.0 450.0 438.0 ... 442.0 458.0 458.0 sur_refl_b02_1 (y, x) float32 3.013e+03 2.809e+03 ... 2.496e+03 2.496e+03 sur_refl_b03_1 (y, x) float32 259.0 235.0 235.0 231.0 ... 230.0 265.0 265.0 sur_refl_b04_1 (y, x) float32 563.0 541.0 541.0 528.0 ... 476.0 518.0 518.0 sur_refl_b07_1 (y, x) float32 832.0 820.0 820.0 820.0 ... 789.0 804.0 804.0 Attributes: (12/136) ADDITIONALLAYERS1KM: 11 ADDITIONALLAYERS500M: 1 ASSOCIATEDINSTRUMENTSHORTNAME.1: MODIS ASSOCIATEDPLATFORMSHORTNAME.1: Terra ASSOCIATEDSENSORSHORTNAME.1: MODIS AUTOMATICQUALITYFLAG.1: Passed ... ... total_additional_observations_1km: 2705510 total_additional_observations_500m: 660129 VERSIONID: 6 VERTICALTILENUMBER: 5 WESTBOUNDINGCOORDINATE: -117.486656023174 ZONEIDENTIFIER: Universal Transverse Mercator UTM
- y: 3
- x: 8
- y(y)float644.446e+06 4.446e+06 4.445e+06
- axis :
- Y
- long_name :
- y coordinate of projection
- standard_name :
- projection_y_coordinate
- units :
- metre
array([4446180.484159, 4445717.171443, 4445253.858726])
- x(x)float64-8.988e+06 ... -8.985e+06
- axis :
- X
- long_name :
- x coordinate of projection
- standard_name :
- projection_x_coordinate
- units :
- metre
array([-8988498.356997, -8988035.04428 , -8987571.731564, -8987108.418847, -8986645.106131, -8986181.793414, -8985718.480698, -8985255.167981])
- band()int641
array(1)
- spatial_ref()int640
- crs_wkt :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- semi_major_axis :
- 6371007.181
- semi_minor_axis :
- 6371007.181
- inverse_flattening :
- 0.0
- reference_ellipsoid_name :
- Custom spheroid
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- Unknown datum based upon the custom spheroid
- horizontal_datum_name :
- Not specified (based on custom spheroid)
- projected_crs_name :
- unnamed
- grid_mapping_name :
- sinusoidal
- longitude_of_projection_origin :
- 0.0
- false_easting :
- 0.0
- false_northing :
- 0.0
- spatial_ref :
- PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not specified (based on custom spheroid)",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["Meter",1],AXIS["Easting",EAST],AXIS["Northing",NORTH]]
- GeoTransform :
- -8988730.01335511 463.3127165277089 0.0 4446412.1405174155 0.0 -463.312716527842
array(0)
- sur_refl_b01_1(y, x)float32428.0 450.0 450.0 ... 458.0 458.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 1 - first layer
- units :
- reflectance
array([[428., 450., 450., 438., 438., 421., 421., 409.], [402., 402., 402., 442., 442., 442., 442., 458.], [402., 402., 442., 442., 442., 442., 458., 458.]], dtype=float32)
- sur_refl_b02_1(y, x)float323.013e+03 2.809e+03 ... 2.496e+03
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 2 - first layer
- units :
- reflectance
array([[3013., 2809., 2809., 2730., 2730., 2628., 2628., 2641.], [2565., 2565., 2565., 2522., 2522., 2522., 2522., 2496.], [2565., 2565., 2522., 2522., 2522., 2522., 2496., 2496.]], dtype=float32)
- sur_refl_b03_1(y, x)float32259.0 235.0 235.0 ... 265.0 265.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 3 - first layer
- units :
- reflectance
array([[259., 235., 235., 231., 231., 211., 211., 203.], [217., 217., 217., 230., 230., 230., 230., 265.], [217., 217., 230., 230., 230., 230., 265., 265.]], dtype=float32)
- sur_refl_b04_1(y, x)float32563.0 541.0 541.0 ... 518.0 518.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 4 - first layer
- units :
- reflectance
array([[563., 541., 541., 528., 528., 494., 494., 486.], [476., 476., 476., 476., 476., 476., 476., 518.], [476., 476., 476., 476., 476., 476., 518., 518.]], dtype=float32)
- sur_refl_b07_1(y, x)float32832.0 820.0 820.0 ... 804.0 804.0
- scale_factor :
- 10000.0
- add_offset :
- 0.0
- long_name :
- 500m Surface Reflectance Band 7 - first layer
- units :
- reflectance
array([[832., 820., 820., 820., 820., 749., 749., 715.], [715., 715., 715., 789., 789., 789., 789., 804.], [715., 715., 789., 789., 789., 789., 804., 804.]], dtype=float32)
- ADDITIONALLAYERS1KM :
- 11
- ADDITIONALLAYERS500M :
- 1
- ASSOCIATEDINSTRUMENTSHORTNAME.1 :
- MODIS
- ASSOCIATEDPLATFORMSHORTNAME.1 :
- Terra
- ASSOCIATEDSENSORSHORTNAME.1 :
- MODIS
- AUTOMATICQUALITYFLAG.1 :
- Passed
- AUTOMATICQUALITYFLAGEXPLANATION.1 :
- No automatic quality assessment is performed in the PGE
- CHARACTERISTICBINANGULARSIZE1KM :
- 30.0
- CHARACTERISTICBINANGULARSIZE500M :
- 15.0
- CHARACTERISTICBINSIZE1KM :
- 926.625433055556
- CHARACTERISTICBINSIZE500M :
- 463.312716527778
- CLOUDOPTION :
- MOD09 internally-derived
- COVERAGECALCULATIONMETHOD :
- volume
- COVERAGEMINIMUM :
- 0.00999999977648258
- DATACOLUMNS1KM :
- 1200
- DATACOLUMNS500M :
- 2400
- DATAROWS1KM :
- 1200
- DATAROWS500M :
- 2400
- DAYNIGHTFLAG :
- Day
- DEEPOCEANFLAG :
- Yes
- DESCRREVISION :
- 6.1
- EASTBOUNDINGCOORDINATE :
- -92.3664205550513
- EQUATORCROSSINGDATE.1 :
- 2016-07-07
- EQUATORCROSSINGDATE.2 :
- 2016-07-07
- EQUATORCROSSINGLONGITUDE.1 :
- -103.273195919522
- EQUATORCROSSINGLONGITUDE.2 :
- -127.994803619317
- EQUATORCROSSINGTIME.1 :
- 17:23:36.891214
- EQUATORCROSSINGTIME.2 :
- 19:02:29.990629
- EXCLUSIONGRINGFLAG.1 :
- N
- FIRSTLAYERSELECTIONCRITERIA :
- order of input pointer
- GEOANYABNORMAL :
- False
- GEOESTMAXRMSERROR :
- 50.0
- GLOBALGRIDCOLUMNS1KM :
- 43200
- GLOBALGRIDCOLUMNS500M :
- 86400
- GLOBALGRIDROWS1KM :
- 21600
- GLOBALGRIDROWS500M :
- 43200
- GRANULEBEGINNINGDATETIME :
- 2016-07-07T17:10:00.000000Z
- GRANULEBEGINNINGDATETIMEARRAY :
- 2016-07-07T17:10:00.000000Z, 2016-07-07T17:15:00.000000Z, 2016-07-07T18:50:00.000000Z, 2016-07-07T20:25:00.000000Z
- GRANULEDAYNIGHTFLAG :
- Day
- GRANULEDAYNIGHTFLAGARRAY :
- Day, Day, Day, Day
- GRANULEDAYOFYEAR :
- 189
- GRANULEENDINGDATETIME :
- 2016-07-07T18:55:00.000000Z
- GRANULEENDINGDATETIMEARRAY :
- 2016-07-07T17:15:00.000000Z, 2016-07-07T17:20:00.000000Z, 2016-07-07T18:55:00.000000Z, 2016-07-07T20:30:00.000000Z
- GRANULENUMBERARRAY :
- 208, 209, 228, 247, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRANULEPOINTERARRAY :
- 0, 1, 2, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- GRINGPOINTLATITUDE.1 :
- 29.8360532722546, 39.9999999964079, 40.0742066197196, 29.9009502428382
- GRINGPOINTLONGITUDE.1 :
- -103.835851753394, -117.486656023174, -104.256722414513, -92.131858571552
- GRINGPOINTSEQUENCENO.1 :
- 1, 2, 3, 4
- HDFEOSVersion :
- HDFEOS_V2.17
- HORIZONTALTILENUMBER :
- 9
- identifier_product_doi :
- 10.5067/MODIS/MOD09GA.006
- identifier_product_doi_authority :
- http://dx.doi.org
- INPUTPOINTER :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf, DEM_SN_H.h09v05.006_0.hdf, MCDLCHKM.A2010001.h09v05.051.2014287174141.hdf
- KEEPALL :
- No
- L2GSTORAGEFORMAT1KM :
- compact
- L2GSTORAGEFORMAT500M :
- compact
- l2g_storage_format_1km :
- compact
- l2g_storage_format_500m :
- compact
- LOCALGRANULEID :
- MOD09GA.A2016189.h09v05.006.2016191073856.hdf
- LOCALVERSIONID :
- 6.0.9
- LONGNAME :
- MODIS/Terra Surface Reflectance Daily L2G Global 1km and 500m SIN Grid
- MAXIMUMOBSERVATIONS1KM :
- 12
- MAXIMUMOBSERVATIONS500M :
- 2
- maximum_observations_1km :
- 12
- maximum_observations_500m :
- 2
- MAXOUTPUTRES :
- QKM
- NADIRDATARESOLUTION1KM :
- 1km
- NADIRDATARESOLUTION500M :
- 500m
- NORTHBOUNDINGCOORDINATE :
- 39.9999999964079
- NumberLandWater1km :
- 0, 1418266, 15655, 6079, 0, 0, 0, 0, 0
- NumberLandWater500m :
- 0, 2836532, 31310, 12158, 0, 0, 0, 0, 0
- NUMBEROFGRANULES :
- 1
- NUMBEROFINPUTGRANULES :
- 4
- NUMBEROFORBITS :
- 2
- NUMBEROFOVERLAPGRANULES :
- 3
- ORBITNUMBER.1 :
- 88050
- ORBITNUMBER.2 :
- 88051
- ORBITNUMBERARRAY :
- 88050, 88050, 88051, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1
- PARAMETERNAME.1 :
- MOD09G
- PERCENTCLOUDY :
- 13
- PERCENTLAND :
- 97
- PERCENTLANDSEAMASKCLASS :
- 0, 97, 3, 0, 0, 0, 0, 0
- PERCENTLOWSUN :
- 0
- PERCENTPROCESSED :
- 100
- PERCENTSHADOW :
- 2
- PGEVERSION :
- 6.0.32
- PROCESSINGCENTER :
- MODAPS
- PROCESSINGENVIRONMENT :
- Linux minion6007 2.6.32-642.1.1.el6.x86_64 #1 SMP Tue May 31 21:57:07 UTC 2016 x86_64 x86_64 x86_64 GNU/Linux
- PROCESSVERSION :
- 6.0.9
- PRODUCTIONDATETIME :
- 2016-07-09T07:38:56.000Z
- QAPERCENTGOODQUALITY :
- 100
- QAPERCENTINTERPOLATEDDATA.1 :
- 0
- QAPERCENTMISSINGDATA.1 :
- 0
- QAPERCENTNOTPRODUCEDCLOUD :
- 0
- QAPERCENTNOTPRODUCEDOTHER :
- 0
- QAPERCENTOTHERQUALITY :
- 0
- QAPERCENTOUTOFBOUNDSDATA.1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND1 :
- 0
- QAPERCENTPOOROUTPUT500MBAND2 :
- 0
- QAPERCENTPOOROUTPUT500MBAND3 :
- 0
- QAPERCENTPOOROUTPUT500MBAND4 :
- 0
- QAPERCENTPOOROUTPUT500MBAND5 :
- 0
- QAPERCENTPOOROUTPUT500MBAND6 :
- 0
- QAPERCENTPOOROUTPUT500MBAND7 :
- 0
- QUALITYCLASSPERCENTAGE500MBAND1 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND2 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND3 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND4 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND5 :
- 96, 0, 0, 0, 0, 0, 0, 0, 4, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND6 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- QUALITYCLASSPERCENTAGE500MBAND7 :
- 100, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0
- RANGEBEGINNINGDATE :
- 2016-07-07
- RANGEBEGINNINGTIME :
- 17:10:00.000000
- RANGEENDINGDATE :
- 2016-07-07
- RANGEENDINGTIME :
- 18:55:00.000000
- RANKING :
- No
- REPROCESSINGACTUAL :
- processed once
- REPROCESSINGPLANNED :
- further update is anticipated
- RESOLUTIONBANDS1AND2 :
- 500
- SCIENCEQUALITYFLAG.1 :
- Not Investigated
- SCIENCEQUALITYFLAGEXPLANATION.1 :
- See http://landweb.nascom.nasa.gov/cgi-bin/QA_WWW/qaFlagPage.cgi?sat=terra for the product Science Quality status.
- SHORTNAME :
- MOD09GA
- SOUTHBOUNDINGCOORDINATE :
- 29.9999999973059
- SPSOPARAMETERS :
- 2015
- SYSTEMFILENAME :
- MOD09GST.A2016189.h09v05.006.2016191073429.hdf, MOD09GHK.A2016189.h09v05.006.2016191073552.hdf, MOD09GQK.A2016189.h09v05.006.2016191073522.hdf, MODPT1KD.A2016189.h09v05.006.2016191073353.hdf, MODPTHKM.A2016189.h09v05.006.2016191073353.hdf, MODPTQKM.A2016189.h09v05.006.2016191073353.hdf, MODMGGAD.A2016189.h09v05.006.2016191073357.hdf, MODTBGD.A2016189.h09v05.006.2016191073601.hdf, MODOCGD.A2016189.h09v05.006.2016191073613.hdf, MOD10L2G.A2016189.h09v05.006.2016191073417.hdf
- TileID :
- 51009005
- TOTALADDITIONALOBSERVATIONS1KM :
- 2705510
- TOTALADDITIONALOBSERVATIONS500M :
- 660129
- TOTALOBSERVATIONS1KM :
- 4145510
- TOTALOBSERVATIONS500M :
- 6420120
- total_additional_observations_1km :
- 2705510
- total_additional_observations_500m :
- 660129
- VERSIONID :
- 6
- VERTICALTILENUMBER :
- 5
- WESTBOUNDINGCOORDINATE :
- -117.486656023174
- ZONEIDENTIFIER :
- Universal Transverse Mercator UTM
The output plot looks similar, yet the processing approach is
different
# View cropped data
f, ax = plt.subplots()
ep.plot_bands(modis_bands_clip.to_array().values[0],
ax=ax,
extent=modis_ext,
title="Plot of the data clipped to the geometry")
fire_bound_sin.plot(ax=ax,
color="purple")
plt.show()
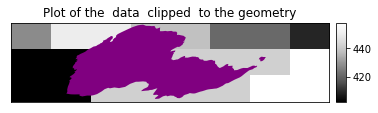
Which Approach To Use?
You may be wondering which of the above scenarios is best. The answer is - it depends. We know that using the crs=
parameter will likely slow down your processing, as well as provide a slightly tilted version of your geometry, however it requires less code to implement.
For small workflows it could be fine to use the approach that uses the least amount of code. For other workflows, you may want to reproject your fire boundary first and use either .clip
(or clip_box) to clip your data.
Hopefully the examples above get your started in the right direction and you can customize your workflow to your science goals and processing needs!
Putting it Together Efficiently
This entire workflow above can be consolidated into an efficient
function. Below is a function that combines many of the items discussed above. Note that this function does incorporate the
crs=
parameter which could slow processing down a bit.
def open_clean_bands(band_path,
crop_layer,
variable=None):
"""Open, subset and crop a MODIS h4 file.
Parameters
-----------
band_path : string
A path to the array to be opened.
crop_layer : geopandas GeoDataFrame
A geopandas dataframe to be used to crop the raster data using rioxarray clip().
variable : List
A list of variables to be opened from the raster.
Returns
-----------
band : xarray DataArray
Cropped xarray DataArray
"""
crop_bound_box = [box(*crop_layer.total_bounds)]
band = rxr.open_rasterio(band_path,
masked=True,
variable=variable).rio.clip(crop_bound_box,
crs=crop_layer.crs,
all_touched=True,
from_disk=True).squeeze()
return band
# Open bands with function
clean_bands = open_clean_bands(band_path=modis_pre_path,
crop_layer=fire_boundary,
variable=desired_bands)
# Plot bands opened with function
ep.plot_bands(clean_bands.to_array().values,
title=desired_bands,
figsize=(10, 4))
plt.show()
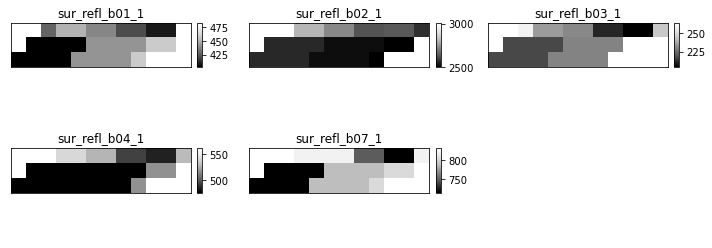
Export MODIS Data as a GeoTIFF
You can quickly export a .tif file using rioxarray
. To
do this you need a path to the new file that includes the new file name. Rioxarray will create a .tif
file with all the correct data and metadata.
# Define path and file name for new tif file
stacked_file_path = os.path.join(os.path.dirname(modis_pre_path),
"final_output",
"modis_band_1.tif")
# Get the directory needed for the defined path
modis_dir_path = os.path.dirname(stacked_file_path)
print("Directory to save path:", modis_dir_path)
# Create the directory if it does not exist
if not os.path.exists(modis_dir_path):
os.mkdir(modis_dir_path)
print("The directory", modis_dir_path, "does not exist - creating it now.")
Directory to save path: cold-springs-modis-h4/07_july_2016/final_output
The directory cold-springs-modis-h4/07_july_2016/final_output does not exist - creating it now.
You can now export or write out a new GeoTIFF file.
# Here you decide how much of the data you want to export.
# A single layer vs a stacked / array
# Export a single band to a geotiff
clean_bands.rio.to_raster(stacked_file_path)
Open and view your stacked GeoTIFF.
# Open the file to make sure it looks ok
modis_b1_xr = rxr.open_rasterio(stacked_file_path,
masked=True)
modis_b1_xr.rio.crs, modis_b1_xr.rio.nodata
(CRS.from_wkt('PROJCS["unnamed",GEOGCS["Unknown datum based upon the custom spheroid",DATUM["Not_specified_based_on_custom_spheroid",SPHEROID["Custom spheroid",6371007.181,0]],PRIMEM["Greenwich",0],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]]],PROJECTION["Sinusoidal"],PARAMETER["longitude_of_center",0],PARAMETER["false_easting",0],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH]]'),
nan)
# Plot the data
ep.plot_bands(modis_b1_xr,
figsize=(10, 4))
plt.show()
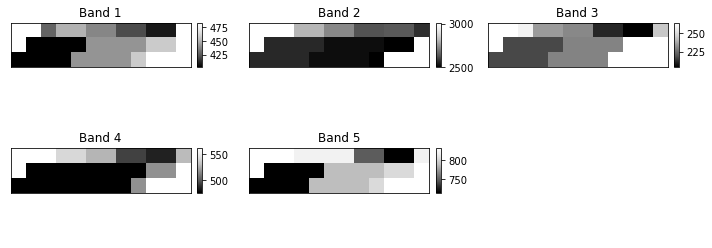
Share on
Twitter Facebook Google+ LinkedIn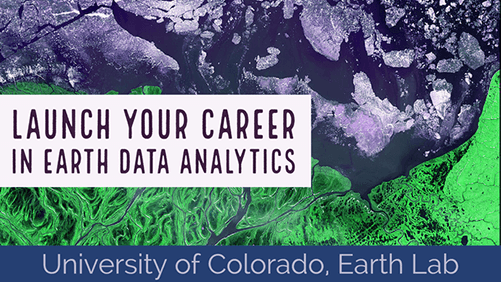
Leave a Comment