Lesson 3. Calculate and Plot Difference Normalized Burn Ratio (dNBR) using Landsat 8 Remote Sensing Data in Python
Learning Objectives
- Calculate
dNBR
in Python.
As discussed in the previous lesson, you can use dNBR to map the extent and severity of a fire. In this lesson, you learn how to create NBR using Landsat data.
You calculate dNBR using the following steps:
- Open up pre-fire data and calculate NBR
- Open up the post-fire data and calculate NBR
- Calculate dNBR (difference NBR) by subtracting post-fire NBR from pre-fire NBR (NBR pre - NBR post fire).
- Classify the dNBR raster using the classification table.
Calculate dNBR Using Landsat Data
First, import your Python libraries.
import os
from glob import glob
import matplotlib.pyplot as plt
from matplotlib import patches as mpatches
from matplotlib.colors import ListedColormap
from matplotlib import colors
import seaborn as sns
import numpy as np
import numpy.ma as ma
from shapely.geometry import mapping, box
from rasterio.plot import plotting_extent
import xarray as xr
import rioxarray as rxr
import geopandas as gpd
import earthpy as et
import earthpy.spatial as es
import earthpy.plot as ep
# Prettier plotting with seaborn
sns.set_style('white')
sns.set(font_scale=1.5)
# Download data and set working directory
data1 = et.data.get_data('cold-springs-fire')
data2 = et.data.get_data('cs-test-landsat')
os.chdir(os.path.join(et.io.HOME,
'earth-analytics',
'data'))
Downloading from https://ndownloader.figshare.com/files/10960214?private_link=fbba903d00e1848b423e
Extracted output to /root/earth-analytics/data/cs-test-landsat/.
To calculate difference Normalized Burn Ratio (dNBR), you first need to calculate NBR for the pre and post fire data. This, of course, presumes that you have data before and after the area was burned from the same remote sensing sensor. Ideally, this data also does not have clouds covering the fire area.
Open up and stack the Landsat post-fire data. You will use the same function that we introduced in the first Landsat lesson.
def combine_tifs(tif_list):
"""A function that combines a list of tifs in the same CRS
and of the same extent into an xarray object
Parameters
----------
tif_list : list
A list of paths to the tif files that you wish to combine.
Returns
-------
An xarray object with all of the tif files in the listmerged into
a single object.
"""
out_xr = []
for i, tif_path in enumerate(tif_list):
out_xr.append(rxr.open_rasterio(tif_path, masked=True).squeeze())
out_xr[i]["band"] = i+1
return xr.concat(out_xr, dim="band")
# Import and stack post fire Landsat data - notice you are only stacking bands 5-7
all_landsat_bands_path = glob(os.path.join("cold-springs-fire",
"landsat_collect",
"LC080340322016072301T1-SC20180214145802",
"crop",
"*band[5-7]*.tif"))
all_landsat_bands_path.sort()
landsat_post_fire = combine_tifs(all_landsat_bands_path)
extent_landsat = plotting_extent(
landsat_post_fire[0].values, landsat_post_fire.rio.transform())
landsat_post_fire
<xarray.DataArray (band: 3, y: 177, x: 246)> array([[[2445., 2271., 2417., ..., 1734., 1904., 2101.], [2662., 2465., 2532., ..., 1736., 1824., 2165.], [2880., 2872., 2750., ..., 1897., 2116., 2300.], ..., [1900., 1917., 2076., ..., 1722., 1891., 1890.], [1779., 1893., 1983., ..., 1645., 1847., 2090.], [1553., 1440., 1587., ..., 1562., 1689., 1964.]], [[2864., 2974., 3108., ..., 983., 1195., 1271.], [2527., 2827., 3008., ..., 1132., 1293., 1546.], [2141., 2427., 2433., ..., 1324., 1652., 1922.], ..., [1662., 1757., 1922., ..., 1463., 1472., 1519.], [1786., 1532., 1554., ..., 1374., 1423., 1450.], [1071., 943., 975., ..., 1524., 1461., 1518.]], [[1920., 1979., 2098., ..., 537., 660., 687.], [1505., 1863., 1975., ..., 651., 747., 924.], [1240., 1407., 1391., ..., 769., 1018., 1189.], ..., [1216., 1190., 1398., ..., 877., 890., 928.], [1517., 1184., 1078., ..., 846., 810., 820.], [ 660., 593., 623., ..., 984., 909., 880.]]], dtype=float32) Coordinates: * band (band) int64 1 2 3 * x (x) float64 4.557e+05 4.557e+05 4.557e+05 ... 4.63e+05 4.63e+05 * y (y) float64 4.428e+06 4.428e+06 ... 4.423e+06 4.423e+06 spatial_ref int64 0 Attributes: STATISTICS_MAXIMUM: 5571 STATISTICS_MEAN: 1958.570001378 STATISTICS_MINIMUM: -2 STATISTICS_STDDEV: 557.005903918 scale_factor: 1.0 add_offset: 0.0
- band: 3
- y: 177
- x: 246
- 2.445e+03 2.271e+03 2.417e+03 2.494e+03 ... 787.0 984.0 909.0 880.0
array([[[2445., 2271., 2417., ..., 1734., 1904., 2101.], [2662., 2465., 2532., ..., 1736., 1824., 2165.], [2880., 2872., 2750., ..., 1897., 2116., 2300.], ..., [1900., 1917., 2076., ..., 1722., 1891., 1890.], [1779., 1893., 1983., ..., 1645., 1847., 2090.], [1553., 1440., 1587., ..., 1562., 1689., 1964.]], [[2864., 2974., 3108., ..., 983., 1195., 1271.], [2527., 2827., 3008., ..., 1132., 1293., 1546.], [2141., 2427., 2433., ..., 1324., 1652., 1922.], ..., [1662., 1757., 1922., ..., 1463., 1472., 1519.], [1786., 1532., 1554., ..., 1374., 1423., 1450.], [1071., 943., 975., ..., 1524., 1461., 1518.]], [[1920., 1979., 2098., ..., 537., 660., 687.], [1505., 1863., 1975., ..., 651., 747., 924.], [1240., 1407., 1391., ..., 769., 1018., 1189.], ..., [1216., 1190., 1398., ..., 877., 890., 928.], [1517., 1184., 1078., ..., 846., 810., 820.], [ 660., 593., 623., ..., 984., 909., 880.]]], dtype=float32)
- band(band)int641 2 3
array([1, 2, 3])
- x(x)float644.557e+05 4.557e+05 ... 4.63e+05
array([455670., 455700., 455730., ..., 462960., 462990., 463020.])
- y(y)float644.428e+06 4.428e+06 ... 4.423e+06
array([4428450., 4428420., 4428390., 4428360., 4428330., 4428300., 4428270., 4428240., 4428210., 4428180., 4428150., 4428120., 4428090., 4428060., 4428030., 4428000., 4427970., 4427940., 4427910., 4427880., 4427850., 4427820., 4427790., 4427760., 4427730., 4427700., 4427670., 4427640., 4427610., 4427580., 4427550., 4427520., 4427490., 4427460., 4427430., 4427400., 4427370., 4427340., 4427310., 4427280., 4427250., 4427220., 4427190., 4427160., 4427130., 4427100., 4427070., 4427040., 4427010., 4426980., 4426950., 4426920., 4426890., 4426860., 4426830., 4426800., 4426770., 4426740., 4426710., 4426680., 4426650., 4426620., 4426590., 4426560., 4426530., 4426500., 4426470., 4426440., 4426410., 4426380., 4426350., 4426320., 4426290., 4426260., 4426230., 4426200., 4426170., 4426140., 4426110., 4426080., 4426050., 4426020., 4425990., 4425960., 4425930., 4425900., 4425870., 4425840., 4425810., 4425780., 4425750., 4425720., 4425690., 4425660., 4425630., 4425600., 4425570., 4425540., 4425510., 4425480., 4425450., 4425420., 4425390., 4425360., 4425330., 4425300., 4425270., 4425240., 4425210., 4425180., 4425150., 4425120., 4425090., 4425060., 4425030., 4425000., 4424970., 4424940., 4424910., 4424880., 4424850., 4424820., 4424790., 4424760., 4424730., 4424700., 4424670., 4424640., 4424610., 4424580., 4424550., 4424520., 4424490., 4424460., 4424430., 4424400., 4424370., 4424340., 4424310., 4424280., 4424250., 4424220., 4424190., 4424160., 4424130., 4424100., 4424070., 4424040., 4424010., 4423980., 4423950., 4423920., 4423890., 4423860., 4423830., 4423800., 4423770., 4423740., 4423710., 4423680., 4423650., 4423620., 4423590., 4423560., 4423530., 4423500., 4423470., 4423440., 4423410., 4423380., 4423350., 4423320., 4423290., 4423260., 4423230., 4423200., 4423170.])
- spatial_ref()int640
- crs_wkt :
- PROJCS["WGS 84 / UTM zone 13N",GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4326"]],PROJECTION["Transverse_Mercator"],PARAMETER["latitude_of_origin",0],PARAMETER["central_meridian",-105],PARAMETER["scale_factor",0.9996],PARAMETER["false_easting",500000],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","32613"]]
- semi_major_axis :
- 6378137.0
- semi_minor_axis :
- 6356752.314245179
- inverse_flattening :
- 298.257223563
- reference_ellipsoid_name :
- WGS 84
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- WGS 84
- horizontal_datum_name :
- World Geodetic System 1984
- projected_crs_name :
- WGS 84 / UTM zone 13N
- grid_mapping_name :
- transverse_mercator
- latitude_of_projection_origin :
- 0.0
- longitude_of_central_meridian :
- -105.0
- false_easting :
- 500000.0
- false_northing :
- 0.0
- scale_factor_at_central_meridian :
- 0.9996
- spatial_ref :
- PROJCS["WGS 84 / UTM zone 13N",GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4326"]],PROJECTION["Transverse_Mercator"],PARAMETER["latitude_of_origin",0],PARAMETER["central_meridian",-105],PARAMETER["scale_factor",0.9996],PARAMETER["false_easting",500000],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","32613"]]
- GeoTransform :
- 455655.0 30.0 0.0 4428465.0 0.0 -30.0
array(0)
- STATISTICS_MAXIMUM :
- 5571
- STATISTICS_MEAN :
- 1958.570001378
- STATISTICS_MINIMUM :
- -2
- STATISTICS_STDDEV :
- 557.005903918
- scale_factor :
- 1.0
- add_offset :
- 0.0
# Open fire boundary layer and reproject it to match the Landsat data
fire_boundary_path = os.path.join("cold-springs-fire",
"vector_layers",
"fire-boundary-geomac",
"co_cold_springs_20160711_2200_dd83.shp")
fire_boundary = gpd.read_file(fire_boundary_path)
# If the CRS are not the same, be sure to reproject
fire_bound_utmz13 = fire_boundary.to_crs(landsat_post_fire.rio.crs)
Next, you can calculate NBR on the post fire data. Remember that NBR uses different bands than NDVI but the calculation formula is the same. For landsat 8 data you will be using bands 7 and 5. And remember because python starts counting at 0 (0-based indexing), and we got just bands 5, 6, 7
in our xarray, that will be indices 2 and 0 when you access them in your numpy array.
Below raster math is used to calculate normalized difference.
# Calculate NBR & plot
landsat_postfire_nbr = (
landsat_post_fire[0]-landsat_post_fire[2]) / (landsat_post_fire[0]+landsat_post_fire[2])
fig, ax = plt.subplots(figsize=(12, 6))
ep.plot_bands(landsat_postfire_nbr,
cmap='PiYG',
vmin=-1,
vmax=1,
ax=ax,
extent=extent_landsat,
title="Landsat derived Normalized Burn Ratio\n 23 July 2016 \n Post Cold Springs Fire")
fire_bound_utmz13.plot(ax=ax,
color='None',
edgecolor='black',
linewidth=2)
plt.show()
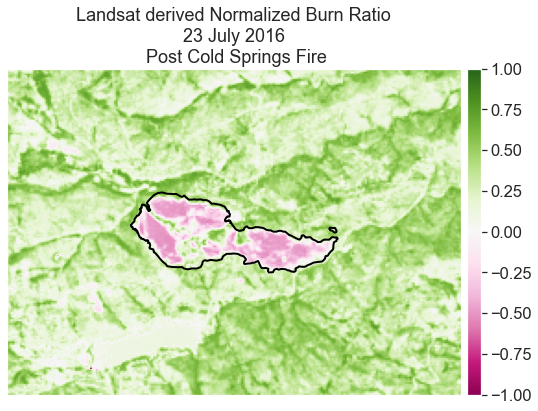
Next, calculate NBR for the pre-fire data. Note that you will have to download the data that is being used below from Earth Explorer following the lessons in this tutorial series.
Also note that you will need to clip or crop the data so that you can subtract the post fire data from the pre fire data. The code to do this is hidden but you did this last week so you should know what to do!
# Are the before and after data the same shape?
landsat_pre_crop.shape == landsat_post_fire.shape
True
Next you can:
- calculate NBR on the pre-fire data and then
- calculate difference dNBR by subtracting the post fire data FROM the pre (pre-post)
The code for this is hidden below because you know how to do this!
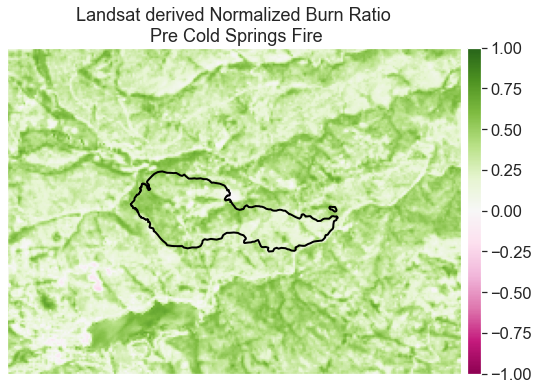
Finally, calculate the difference between the two rasters to calculate the Difference Normalized Burn Ratio (dNBR). Remember to subtract post fire from pre fire.
# Calculate dnbr
dnbr_landsat = nbr_landsat_pre_fire - landsat_postfire_nbr
dnbr_landsat
<xarray.DataArray (y: 177, x: 246)> array([[ 0.04334395, 0.08738004, 0.08557479, ..., -0.04640323, -0.04672405, -0.02588046], [-0.03804424, 0.0463331 , 0.06357773, ..., -0.02489722, -0.01969054, -0.02097243], [-0.06424496, -0.00296727, 0.01671243, ..., -0.03296438, -0.01451224, -0.01793674], ..., [-0.0817215 , -0.01774661, -0.00354043, ..., -0.00419807, 0.01162347, 0.01833299], [ 0.01980466, -0.14882201, -0.06864975, ..., -0.02433777, -0.02668497, -0.02546719], [-0.12681231, 0.00381416, -0.05303624, ..., 0.0295179 , -0.00804126, -0.03982082]], dtype=float32) Coordinates: * y (y) float64 4.428e+06 4.428e+06 ... 4.423e+06 4.423e+06 * x (x) float64 4.557e+05 4.557e+05 4.557e+05 ... 4.63e+05 4.63e+05 spatial_ref int64 0
- y: 177
- x: 246
- 0.04334 0.08738 0.08557 0.08182 ... 0.02952 -0.008041 -0.03982
array([[ 0.04334395, 0.08738004, 0.08557479, ..., -0.04640323, -0.04672405, -0.02588046], [-0.03804424, 0.0463331 , 0.06357773, ..., -0.02489722, -0.01969054, -0.02097243], [-0.06424496, -0.00296727, 0.01671243, ..., -0.03296438, -0.01451224, -0.01793674], ..., [-0.0817215 , -0.01774661, -0.00354043, ..., -0.00419807, 0.01162347, 0.01833299], [ 0.01980466, -0.14882201, -0.06864975, ..., -0.02433777, -0.02668497, -0.02546719], [-0.12681231, 0.00381416, -0.05303624, ..., 0.0295179 , -0.00804126, -0.03982082]], dtype=float32)
- y(y)float644.428e+06 4.428e+06 ... 4.423e+06
- axis :
- Y
- long_name :
- y coordinate of projection
- standard_name :
- projection_y_coordinate
- units :
- metre
array([4428450., 4428420., 4428390., 4428360., 4428330., 4428300., 4428270., 4428240., 4428210., 4428180., 4428150., 4428120., 4428090., 4428060., 4428030., 4428000., 4427970., 4427940., 4427910., 4427880., 4427850., 4427820., 4427790., 4427760., 4427730., 4427700., 4427670., 4427640., 4427610., 4427580., 4427550., 4427520., 4427490., 4427460., 4427430., 4427400., 4427370., 4427340., 4427310., 4427280., 4427250., 4427220., 4427190., 4427160., 4427130., 4427100., 4427070., 4427040., 4427010., 4426980., 4426950., 4426920., 4426890., 4426860., 4426830., 4426800., 4426770., 4426740., 4426710., 4426680., 4426650., 4426620., 4426590., 4426560., 4426530., 4426500., 4426470., 4426440., 4426410., 4426380., 4426350., 4426320., 4426290., 4426260., 4426230., 4426200., 4426170., 4426140., 4426110., 4426080., 4426050., 4426020., 4425990., 4425960., 4425930., 4425900., 4425870., 4425840., 4425810., 4425780., 4425750., 4425720., 4425690., 4425660., 4425630., 4425600., 4425570., 4425540., 4425510., 4425480., 4425450., 4425420., 4425390., 4425360., 4425330., 4425300., 4425270., 4425240., 4425210., 4425180., 4425150., 4425120., 4425090., 4425060., 4425030., 4425000., 4424970., 4424940., 4424910., 4424880., 4424850., 4424820., 4424790., 4424760., 4424730., 4424700., 4424670., 4424640., 4424610., 4424580., 4424550., 4424520., 4424490., 4424460., 4424430., 4424400., 4424370., 4424340., 4424310., 4424280., 4424250., 4424220., 4424190., 4424160., 4424130., 4424100., 4424070., 4424040., 4424010., 4423980., 4423950., 4423920., 4423890., 4423860., 4423830., 4423800., 4423770., 4423740., 4423710., 4423680., 4423650., 4423620., 4423590., 4423560., 4423530., 4423500., 4423470., 4423440., 4423410., 4423380., 4423350., 4423320., 4423290., 4423260., 4423230., 4423200., 4423170.])
- x(x)float644.557e+05 4.557e+05 ... 4.63e+05
- axis :
- X
- long_name :
- x coordinate of projection
- standard_name :
- projection_x_coordinate
- units :
- metre
array([455670., 455700., 455730., ..., 462960., 462990., 463020.])
- spatial_ref()int640
- crs_wkt :
- PROJCS["WGS 84 / UTM zone 13N",GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4326"]],PROJECTION["Transverse_Mercator"],PARAMETER["latitude_of_origin",0],PARAMETER["central_meridian",-105],PARAMETER["scale_factor",0.9996],PARAMETER["false_easting",500000],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","32613"]]
- semi_major_axis :
- 6378137.0
- semi_minor_axis :
- 6356752.314245179
- inverse_flattening :
- 298.257223563
- reference_ellipsoid_name :
- WGS 84
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- WGS 84
- horizontal_datum_name :
- World Geodetic System 1984
- projected_crs_name :
- WGS 84 / UTM zone 13N
- grid_mapping_name :
- transverse_mercator
- latitude_of_projection_origin :
- 0.0
- longitude_of_central_meridian :
- -105.0
- false_easting :
- 500000.0
- false_northing :
- 0.0
- scale_factor_at_central_meridian :
- 0.9996
- spatial_ref :
- PROJCS["WGS 84 / UTM zone 13N",GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4326"]],PROJECTION["Transverse_Mercator"],PARAMETER["latitude_of_origin",0],PARAMETER["central_meridian",-105],PARAMETER["scale_factor",0.9996],PARAMETER["false_easting",500000],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","32613"]]
- GeoTransform :
- 455655.0 30.0 0.0 4428465.0 0.0 -30.0
array(0)
Finally you can classify the data. Remember that dNBR has a set of classification bins and associated categories that are commonly used. When you have calculated NBR - classify the output raster using the np.digitize()
function. Use the dNBR classes below.
SEVERITY LEVEL | dNBR RANGE | |
---|---|---|
Enhanced Regrowth | > -.1 | |
Unburned | -.1 to + .1 | |
Low Severity | +.1 to +.27 | |
Moderate Severity | +.27 to +.66 | |
High Severity | > +.66 |
NOTE: your min and max values for NBR may be slightly different from the table shown above. In the code example above, np.inf
is used to indicate any values larger than .66
.
The code to classify below is hidden! You learned how to classify rasters in week 2 of this course.
Data Tip: You learned how to classify rasters in the lidar raster lessons
# Define dNBR classification bins
dnbr_class_bins = [-np.inf, -.1, .1, .27, .66, np.inf]
#dnbr_landsat_class = np.digitize(dnbr_landsat, dnbr_class_bins)
dnbr_landsat_class = xr.apply_ufunc(np.digitize,
dnbr_landsat,
dnbr_class_bins)
dnbr_landsat_class
<xarray.DataArray (y: 177, x: 246)> array([[2, 2, 2, ..., 2, 2, 2], [2, 2, 2, ..., 2, 2, 2], [2, 2, 2, ..., 2, 2, 2], ..., [2, 2, 2, ..., 2, 2, 2], [2, 1, 2, ..., 2, 2, 2], [1, 2, 2, ..., 2, 2, 2]]) Coordinates: * y (y) float64 4.428e+06 4.428e+06 ... 4.423e+06 4.423e+06 * x (x) float64 4.557e+05 4.557e+05 4.557e+05 ... 4.63e+05 4.63e+05 spatial_ref int64 0
- y: 177
- x: 246
- 2 2 2 2 2 2 2 2 3 2 2 3 3 3 2 2 2 ... 2 2 2 2 2 2 2 2 2 2 2 2 2 2 2 2
array([[2, 2, 2, ..., 2, 2, 2], [2, 2, 2, ..., 2, 2, 2], [2, 2, 2, ..., 2, 2, 2], ..., [2, 2, 2, ..., 2, 2, 2], [2, 1, 2, ..., 2, 2, 2], [1, 2, 2, ..., 2, 2, 2]])
- y(y)float644.428e+06 4.428e+06 ... 4.423e+06
- axis :
- Y
- long_name :
- y coordinate of projection
- standard_name :
- projection_y_coordinate
- units :
- metre
array([4428450., 4428420., 4428390., 4428360., 4428330., 4428300., 4428270., 4428240., 4428210., 4428180., 4428150., 4428120., 4428090., 4428060., 4428030., 4428000., 4427970., 4427940., 4427910., 4427880., 4427850., 4427820., 4427790., 4427760., 4427730., 4427700., 4427670., 4427640., 4427610., 4427580., 4427550., 4427520., 4427490., 4427460., 4427430., 4427400., 4427370., 4427340., 4427310., 4427280., 4427250., 4427220., 4427190., 4427160., 4427130., 4427100., 4427070., 4427040., 4427010., 4426980., 4426950., 4426920., 4426890., 4426860., 4426830., 4426800., 4426770., 4426740., 4426710., 4426680., 4426650., 4426620., 4426590., 4426560., 4426530., 4426500., 4426470., 4426440., 4426410., 4426380., 4426350., 4426320., 4426290., 4426260., 4426230., 4426200., 4426170., 4426140., 4426110., 4426080., 4426050., 4426020., 4425990., 4425960., 4425930., 4425900., 4425870., 4425840., 4425810., 4425780., 4425750., 4425720., 4425690., 4425660., 4425630., 4425600., 4425570., 4425540., 4425510., 4425480., 4425450., 4425420., 4425390., 4425360., 4425330., 4425300., 4425270., 4425240., 4425210., 4425180., 4425150., 4425120., 4425090., 4425060., 4425030., 4425000., 4424970., 4424940., 4424910., 4424880., 4424850., 4424820., 4424790., 4424760., 4424730., 4424700., 4424670., 4424640., 4424610., 4424580., 4424550., 4424520., 4424490., 4424460., 4424430., 4424400., 4424370., 4424340., 4424310., 4424280., 4424250., 4424220., 4424190., 4424160., 4424130., 4424100., 4424070., 4424040., 4424010., 4423980., 4423950., 4423920., 4423890., 4423860., 4423830., 4423800., 4423770., 4423740., 4423710., 4423680., 4423650., 4423620., 4423590., 4423560., 4423530., 4423500., 4423470., 4423440., 4423410., 4423380., 4423350., 4423320., 4423290., 4423260., 4423230., 4423200., 4423170.])
- x(x)float644.557e+05 4.557e+05 ... 4.63e+05
- axis :
- X
- long_name :
- x coordinate of projection
- standard_name :
- projection_x_coordinate
- units :
- metre
array([455670., 455700., 455730., ..., 462960., 462990., 463020.])
- spatial_ref()int640
- crs_wkt :
- PROJCS["WGS 84 / UTM zone 13N",GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4326"]],PROJECTION["Transverse_Mercator"],PARAMETER["latitude_of_origin",0],PARAMETER["central_meridian",-105],PARAMETER["scale_factor",0.9996],PARAMETER["false_easting",500000],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","32613"]]
- semi_major_axis :
- 6378137.0
- semi_minor_axis :
- 6356752.314245179
- inverse_flattening :
- 298.257223563
- reference_ellipsoid_name :
- WGS 84
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- WGS 84
- horizontal_datum_name :
- World Geodetic System 1984
- projected_crs_name :
- WGS 84 / UTM zone 13N
- grid_mapping_name :
- transverse_mercator
- latitude_of_projection_origin :
- 0.0
- longitude_of_central_meridian :
- -105.0
- false_easting :
- 500000.0
- false_northing :
- 0.0
- scale_factor_at_central_meridian :
- 0.9996
- spatial_ref :
- PROJCS["WGS 84 / UTM zone 13N",GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4326"]],PROJECTION["Transverse_Mercator"],PARAMETER["latitude_of_origin",0],PARAMETER["central_meridian",-105],PARAMETER["scale_factor",0.9996],PARAMETER["false_easting",500000],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","32613"]]
- GeoTransform :
- 455655.0 30.0 0.0 4428465.0 0.0 -30.0
array(0)
Finally you are ready to plot your data. Note that legends in python can be tricky so you are provided with two legend options below. In the first plot, you create a legend with individual boxes. Creating a legend this way forces you to use matplotlib patches. Effectively you are drawing each box to create a legend from colors you’ve used in your image.
dnbr_cat_names = ["Enhanced Regrowth",
"Unburned",
"Low Severity",
"Moderate Severity",
"High Severity"]
nbr_colors = ["g",
"yellowgreen",
"peachpuff",
"coral",
"maroon"]
nbr_cmap = ListedColormap(nbr_colors)
Once you setup the legend with
- a ListedColormap: this is a discrete colormap. Thus it will only contain
n
colors rather than a gradient of colors. - A list of titles for each category in your classification scheme: this is what will be “written” on your legend
You are ready to plot. Below you add a custom legend with unique boxes for each class. This is one way to create a legend. It requires a bit more knowledge of matplotlib under the hood to make this work!
# Plot the data with a custom legend
dnbr_landsat_class_plot = ma.masked_array(
dnbr_landsat_class.values, dnbr_landsat_class.isnull())
fig, ax = plt.subplots(figsize=(10, 8))
fire_bound_utmz13.plot(ax=ax,
color='None',
edgecolor='black',
linewidth=2)
classes = np.unique(dnbr_landsat_class_plot)
classes = classes.tolist()[:5]
ep.plot_bands(dnbr_landsat_class_plot,
cmap=nbr_cmap,
vmin=1,
vmax=5,
title="Landsat dNBR - Cold Spring Fire Site \n June 22, 2016 - July 24, 2016",
cbar=False,
scale=False,
extent=extent_landsat,
ax=ax)
ep.draw_legend(im_ax=ax.get_images()[0],
classes=classes,
titles=dnbr_cat_names)
plt.show()
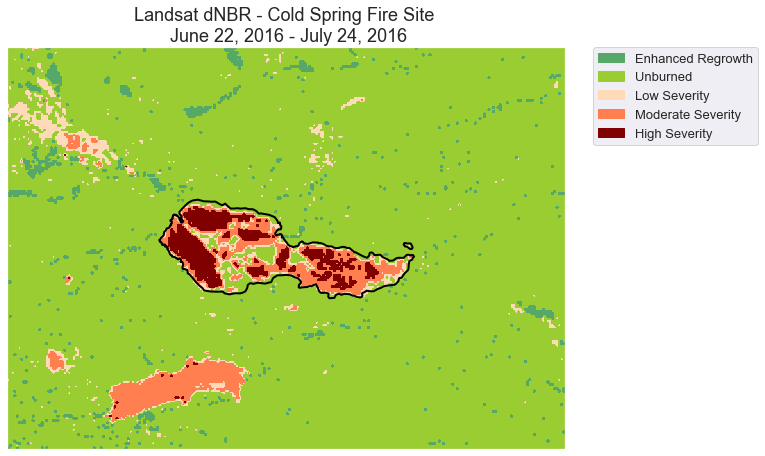
Create a Colorbar Legend - BONUS!
You can also create a discrete colorbar with labels. This method might be a bit less technical to follow. You can decide what type of legend you prefer for your homework.
# Grab raster unique values (classes)
values = np.unique(dnbr_landsat_class).tolist()
# Add another index value because for n categories
# you need n+1 values to create bins
values = [0] + values
# Make a color map of fixed colors
nbr_colors = ["g", "yellowgreen", "peachpuff", "coral", "maroon"]
nbr_cmap = ListedColormap(nbr_colors)
# But the goal is the identify the MIDDLE point
# of each bin to create a centered tick
bounds = [((a + b) / 2) for a, b in zip(values[:-1], values[1::1])] + [5.5]
# Define normalization
norm = colors.BoundaryNorm(bounds, nbr_cmap.N)
Then, plot the data but this time add a colorbar rather than a custom legend.
# Plot the data
fig, ax = plt.subplots(figsize=(10, 8))
ep.plot_bands(dnbr_landsat_class,
ax=ax,
cmap=nbr_cmap,
norm=norm,
cbar=False)
cbar = ep.colorbar(ax.get_images()[0])
cbar.set_ticks([np.unique(dnbr_landsat_class)])
cbar.set_ticklabels(dnbr_cat_names)
ax.set_title("Landsat dNBR - Cold Spring Fire Site \n June 22, 2017 - July 24, 2017",
fontsize=16)
# Turn off ticks
ax.set_axis_off()
plt.show()
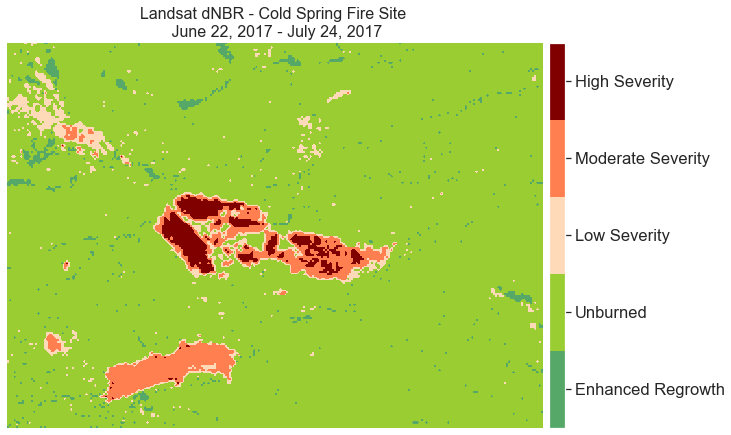
Calculate Total Area of Burned Area
Once you have classified your data, you can calculate the total burn area.
For this lesson, you are comparing the area calculated using modis that is “high severity” to that of Landsat.
You can calculate this using a loop, a function or manually - like so:
# To calculate area, multiply:
# number of pixels in each bin by image resolution
# Result will be in total square meters
landsat_pixel = landsat_pre_crop.rio.resolution(
)[0] * landsat_pre_crop.rio.resolution()[0]
burned_landsat = (dnbr_landsat_class == 5).sum()
burned_landsat = np.multiply(burned_landsat, landsat_pixel).values
print("Landsat Severe Burn Area:", burned_landsat)
Landsat Severe Burn Area: 711900.0
Export dNBR Raster to Geotiff
Finally you can export the newly created dNBR raster to a geotiff.
You can export the data like we exported other rasters. However, an issue you’ll run into with dNBR. np.digitize
creates an array full of int64
values. These values use a lot of memory, so rio.to_raster
doesn’t allow them to be exported. To fix this, you can set the exporting datatype to be int8
, which uses significantly less data. (int32
, float32
, int16
, and more datatypes would work as well, int8
works because all the numbers in this array are relatively small.)
# Create a path to export the data too
dnbr_path = os.path.join("cold-springs-fire", "outputs", "dnbr_landsat.tif")
# Export the data as int8
dnbr_landsat_class.rio.to_raster(dnbr_path, dtype="int8")
# Open and view the exported data
# Note the default datatype to open a raster as is float32!
rxr.open_rasterio(dnbr_path).squeeze()
<xarray.DataArray (y: 177, x: 246)> array([[2, 2, 2, ..., 2, 2, 2], [2, 2, 2, ..., 2, 2, 2], [2, 2, 2, ..., 2, 2, 2], ..., [2, 2, 2, ..., 2, 2, 2], [2, 1, 2, ..., 2, 2, 2], [1, 2, 2, ..., 2, 2, 2]], dtype=int8) Coordinates: band int64 1 * x (x) float64 4.557e+05 4.557e+05 4.557e+05 ... 4.63e+05 4.63e+05 * y (y) float64 4.428e+06 4.428e+06 ... 4.423e+06 4.423e+06 spatial_ref int64 0 Attributes: scale_factor: 1.0 add_offset: 0.0
- y: 177
- x: 246
- 2 2 2 2 2 2 2 2 3 2 2 3 3 3 2 2 2 ... 2 2 2 2 2 2 2 2 2 2 2 2 2 2 2 2
array([[2, 2, 2, ..., 2, 2, 2], [2, 2, 2, ..., 2, 2, 2], [2, 2, 2, ..., 2, 2, 2], ..., [2, 2, 2, ..., 2, 2, 2], [2, 1, 2, ..., 2, 2, 2], [1, 2, 2, ..., 2, 2, 2]], dtype=int8)
- band()int641
array(1)
- x(x)float644.557e+05 4.557e+05 ... 4.63e+05
array([455670., 455700., 455730., ..., 462960., 462990., 463020.])
- y(y)float644.428e+06 4.428e+06 ... 4.423e+06
array([4428450., 4428420., 4428390., 4428360., 4428330., 4428300., 4428270., 4428240., 4428210., 4428180., 4428150., 4428120., 4428090., 4428060., 4428030., 4428000., 4427970., 4427940., 4427910., 4427880., 4427850., 4427820., 4427790., 4427760., 4427730., 4427700., 4427670., 4427640., 4427610., 4427580., 4427550., 4427520., 4427490., 4427460., 4427430., 4427400., 4427370., 4427340., 4427310., 4427280., 4427250., 4427220., 4427190., 4427160., 4427130., 4427100., 4427070., 4427040., 4427010., 4426980., 4426950., 4426920., 4426890., 4426860., 4426830., 4426800., 4426770., 4426740., 4426710., 4426680., 4426650., 4426620., 4426590., 4426560., 4426530., 4426500., 4426470., 4426440., 4426410., 4426380., 4426350., 4426320., 4426290., 4426260., 4426230., 4426200., 4426170., 4426140., 4426110., 4426080., 4426050., 4426020., 4425990., 4425960., 4425930., 4425900., 4425870., 4425840., 4425810., 4425780., 4425750., 4425720., 4425690., 4425660., 4425630., 4425600., 4425570., 4425540., 4425510., 4425480., 4425450., 4425420., 4425390., 4425360., 4425330., 4425300., 4425270., 4425240., 4425210., 4425180., 4425150., 4425120., 4425090., 4425060., 4425030., 4425000., 4424970., 4424940., 4424910., 4424880., 4424850., 4424820., 4424790., 4424760., 4424730., 4424700., 4424670., 4424640., 4424610., 4424580., 4424550., 4424520., 4424490., 4424460., 4424430., 4424400., 4424370., 4424340., 4424310., 4424280., 4424250., 4424220., 4424190., 4424160., 4424130., 4424100., 4424070., 4424040., 4424010., 4423980., 4423950., 4423920., 4423890., 4423860., 4423830., 4423800., 4423770., 4423740., 4423710., 4423680., 4423650., 4423620., 4423590., 4423560., 4423530., 4423500., 4423470., 4423440., 4423410., 4423380., 4423350., 4423320., 4423290., 4423260., 4423230., 4423200., 4423170.])
- spatial_ref()int640
- crs_wkt :
- PROJCS["WGS 84 / UTM zone 13N",GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4326"]],PROJECTION["Transverse_Mercator"],PARAMETER["latitude_of_origin",0],PARAMETER["central_meridian",-105],PARAMETER["scale_factor",0.9996],PARAMETER["false_easting",500000],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","32613"]]
- semi_major_axis :
- 6378137.0
- semi_minor_axis :
- 6356752.314245179
- inverse_flattening :
- 298.257223563
- reference_ellipsoid_name :
- WGS 84
- longitude_of_prime_meridian :
- 0.0
- prime_meridian_name :
- Greenwich
- geographic_crs_name :
- WGS 84
- horizontal_datum_name :
- World Geodetic System 1984
- projected_crs_name :
- WGS 84 / UTM zone 13N
- grid_mapping_name :
- transverse_mercator
- latitude_of_projection_origin :
- 0.0
- longitude_of_central_meridian :
- -105.0
- false_easting :
- 500000.0
- false_northing :
- 0.0
- scale_factor_at_central_meridian :
- 0.9996
- spatial_ref :
- PROJCS["WGS 84 / UTM zone 13N",GEOGCS["WGS 84",DATUM["WGS_1984",SPHEROID["WGS 84",6378137,298.257223563,AUTHORITY["EPSG","7030"]],AUTHORITY["EPSG","6326"]],PRIMEM["Greenwich",0,AUTHORITY["EPSG","8901"]],UNIT["degree",0.0174532925199433,AUTHORITY["EPSG","9122"]],AUTHORITY["EPSG","4326"]],PROJECTION["Transverse_Mercator"],PARAMETER["latitude_of_origin",0],PARAMETER["central_meridian",-105],PARAMETER["scale_factor",0.9996],PARAMETER["false_easting",500000],PARAMETER["false_northing",0],UNIT["metre",1,AUTHORITY["EPSG","9001"]],AXIS["Easting",EAST],AXIS["Northing",NORTH],AUTHORITY["EPSG","32613"]]
- GeoTransform :
- 455655.0 30.0 0.0 4428465.0 0.0 -30.0
array(0)
- scale_factor :
- 1.0
- add_offset :
- 0.0
Share on
Twitter Facebook Google+ LinkedIn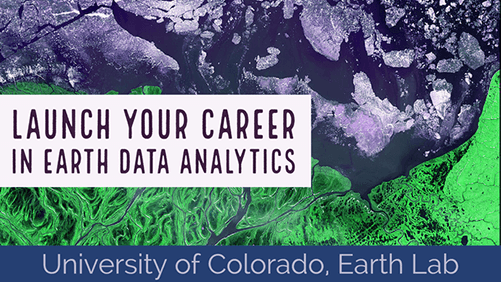
Leave a Comment