Lesson 4. Create interactive leaflet maps using folium in Jupyter Notebook: GIS in Python
Learning Objectives
- Create interactive plots of vector data using folium in Python and Jupyter Notebook.
Create Interactive Map
Begin by importing the necessary packages including geopandas to import the vector data and folium to create the interactive map.
import os
import folium
import geopandas as gpd
import earthpy as et
# Get the data and set working directory
data = et.data.get_data('spatial-vector-lidar')
os.chdir(os.path.join(et.io.HOME, 'earth-analytics', 'data'))
# Create interactive map with default basemap
map_osm = folium.Map(location=[45.5236, -122.6750])
map_osm
0 -119.73765
Name: minx, dtype: float64
/tmp/ipykernel_1870/30331417.py:15: UserWarning: Geometry is in a geographic CRS. Results from 'centroid' are likely incorrect. Use 'GeoSeries.to_crs()' to re-project geometries to a projected CRS before this operation.
mapcen = sjer_crop_extent["geometry"].centroid
# Import SJER plot locations using geopandas
SJER_plot_locations_path = os.path.join("spatial-vector-lidar",
"california",
"neon-sjer-site",
"vector_data",
"SJER_plot_centroids.shp")
SJER_plot_locations = gpd.read_file(SJER_plot_locations_path)
Add Vector Data to Interactive Map
# Project to WGS 84 and save to json for plotting on interactive map
SJER_plot_locations_json = SJER_plot_locations.to_crs(epsg=4326).to_json()
# Create interactive map and add SJER plot locations
SJER_map = folium.Map([37.12, -119.737],
zoom_start=14)
points = folium.features.GeoJson(SJER_plot_locations_json)
SJER_map.add_child(points)
SJER_map
# Create interactive map with different basemap
SJER_map = folium.Map([37.12, -119.737],
zoom_start=14,
tiles='Stamen Terrain')
points = folium.features.GeoJson(SJER_plot_locations_json)
SJER_map.add_child(points)
SJER_map
Share on
Twitter Facebook Google+ LinkedIn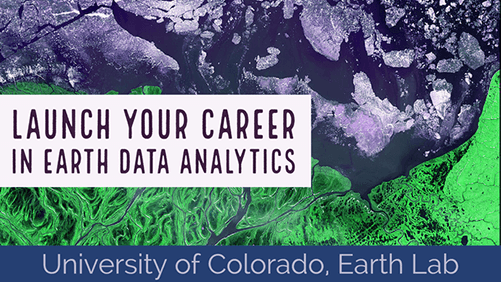
Leave a Comment